第一行和第二行分别输入两个字符串s1和s2,第三行输入插入位置f。 每个字符串的长度不超过100个字符。 输出一行插入后的字符串。
时间: 2024-11-22 13:36:43 浏览: 29
这是一个简单的控制台程序设计任务,可以使用C语言基本的输入和输出函数来完成。以下是一个例子:
```c
#include <stdio.h>
#include <string.h>
// 函数用于插入字符串
void insert_string(int f, char* s1, char* s2) {
// 检查输入是否合法
if (f <= 0 || strlen(s1) > 100 || strlen(s2) > 100) {
printf("Invalid input! String lengths should not exceed 100 characters and insertion position must be positive.\n");
return;
}
// 利用strncat函数在s1的第f个位置开始插入s2
char result[101]; // 足够存放最大100字符的字符串和一个结束符
strncat(result, s1, f); // 保留原始的s1前f个字符
// 插入s2
strncat(result, s2, strlen(s2));
// 结束符
result[strlen(result)] = '\0';
printf("Inserted string: %s\n", result);
}
int main() {
char s1[101], s2[101];
int f;
// 输入字符串和插入位置
printf("Enter the first string (up to 100 chars): ");
fgets(s1, sizeof(s1), stdin);
s1[strcspn(s1, "\n")] = '\0'; // 移除fgets获取的换行符
printf("Enter the second string (up to 100 chars): ");
fgets(s2, sizeof(s2), stdin);
s2[strcspn(s2, "\n")] = '\0'; // 移除fgets获取的换行符
printf("Enter the insertion position: ");
scanf("%d", &f); // 防止输入非法字符,此处假定用户只输入数字
// 调用函数插入字符串
insert_string(f, s1, s2);
return 0;
}
```
当运行这个程序时,它会提示用户输入字符串和插入位置,然后按照提供的信息输出插入后的字符串。
阅读全文
相关推荐
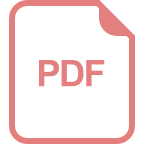
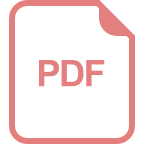
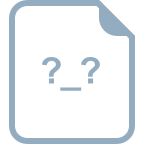















