Java完成一个企业人事管理系统,该系统中包含两个实体类:员工(Emp),部门(Dept),两个类中分别包含以下属性: 员工(工号,姓名,性别,职位,年龄,月薪,部门) 部门(编号,部门名,部门介绍,分机号) 要求实现以下功能: 完成部门的创建,添加3个部门 向各个部门中添加若干员工 查询所有的员工信息和所在的部门信息 根据员工工号显示员工信息 修改指定工号员工的薪资 根据部门号查询出门中的所有员工
时间: 2024-04-12 15:31:26 浏览: 23
下面是一个简单的 Java 代码示例,用于实现企业人事管理系统中的员工和部门功能。请注意,以下示例仅供参考,你可以根据自己的需求进行修改和扩展。
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Scanner;
class Employee {
private int id;
private String name;
private String gender;
private String position;
private int age;
private double salary;
private int departmentId;
public Employee(int id, String name, String gender, String position, int age, double salary, int departmentId) {
this.id = id;
this.name = name;
this.gender = gender;
this.position = position;
this.age = age;
this.salary = salary;
this.departmentId = departmentId;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public String getPosition() {
return position;
}
public int getAge() {
return age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public int getDepartmentId() {
return departmentId;
}
}
class Department {
private int id;
private String name;
private String description;
private int extensionNumber;
public Department(int id, String name, String description, int extensionNumber) {
this.id = id;
this.name = name;
this.description = description;
this.extensionNumber = extensionNumber;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getDescription() {
return description;
}
public int getExtensionNumber() {
return extensionNumber;
}
}
class HRManagementSystem {
private List<Employee> employees;
private Map<Integer, Department> departments;
public HRManagementSystem() {
employees = new ArrayList<>();
departments = new HashMap<>();
}
public void createDepartment(int id, String name, String description, int extensionNumber) {
Department department = new Department(id, name, description, extensionNumber);
departments.put(id, department);
System.out.println("部门创建成功!");
}
public void addEmployee(Employee employee) {
employees.add(employee);
System.out.println("员工添加成功!");
}
public void displayAllEmployees() {
if (employees.isEmpty()) {
System.out.println("暂无员工信息!");
} else {
System.out.println("所有员工信息:");
for (Employee employee : employees) {
System.out.println("工号:" + employee.getId());
System.out.println("姓名:" + employee.getName());
System.out.println("性别:" + employee.getGender());
System.out.println("职位:" + employee.getPosition());
System.out.println("年龄:" + employee.getAge());
System.out.println("月薪:" + employee.getSalary());
int departmentId = employee.getDepartmentId();
Department department = departments.get(departmentId);
System.out.println("部门编号:" + department.getId());
System.out.println("部门名称:" + department.getName());
System.out.println("--------------------");
}
}
}
public void displayEmployeeById(int id) {
for (Employee employee : employees) {
if (employee.getId() == id) {
System.out.println("工号:" + employee.getId());
System.out.println("姓名:" + employee.getName());
System.out.println("性别:" + employee.getGender());
System.out.println("职位:" + employee.getPosition());
System.out.println("年龄:" + employee.getAge());
System.out.println("月薪:" + employee.getSalary());
int departmentId = employee.getDepartmentId();
Department department = departments.get(departmentId);
System.out.println("部门编号:" + department.getId());
System.out.println("部门名称:" + department.getName());
return;
}
}
System.out.println("找不到指定工号的员工!");
}
public void modifyEmployeeSalary(int id, double newSalary) {
for (Employee employee : employees) {
if (employee.getId() == id) {
employee.setSalary(newSalary);
System.out.println("员工薪资修改成功!");
return;
}
}
System.out.println("找不到指定工号的员工!");
}
public void displayEmployeesByDepartmentId(int departmentId) {
if (!departments.containsKey(departmentId)) {
System.out.println("找不到指定部门!");
return;
}
System.out.println("部门编号:" + departmentId);
Department department = departments.get(departmentId);
System.out.println("部门名称:" + department.getName());
System.out.println("部门介绍:" + department.getDescription());
System.out.println("分机号:" + department.getExtensionNumber());
List<Employee> departmentEmployees = new ArrayList<>();
for (Employee employee : employees) {
if (employee.getDepartmentId() == departmentId) {
departmentEmployees.add(employee);
}
}
if (departmentEmployees.isEmpty()) {
System.out.println("该部门暂无员工!");
} else {
System.out.println("部门员工信息:");
for (Employee employee : departmentEmployees) {
System.out.println("工号:" + employee.getId());
System.out.println("姓名:" + employee.getName());
System.out.println("性别:" + employee.getGender());
System.out.println("职位:" + employee.getPosition());
System.out.println("年龄:" + employee.getAge());
System.out.println("月薪:" + employee.getSalary());
System.out.println("--------------------");
}
}
}
}
public class Main {
public static void main(String[] args) {
HRManagementSystem system = new HRManagementSystem();
Scanner scanner = new Scanner(System.in);
// 创建部门
system.createDepartment(1, "技术部", "负责公司技术开发", 1001);
system.createDepartment(2, "市场部", "负责公司市场拓展", 1002);
system.createDepartment(3, "人事部", "负责公司人力资源管理", 1003);
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加员工");
System.out.println("2. 查询所有员工信息");
System.out.println("3. 根据员工工号显示员工信息");
System.out.println("4. 修改指定工号员工的薪资");
System.out.println("5. 根据部门号查询部门中的员工");
System.out.println("0. 退出程序");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入员工信息:");
System.out.print("工号:");
int id = scanner.nextInt();
scanner.nextLine(); // 消耗换行符
System.out.print("姓名:");
String name = scanner.nextLine();
System.out.print("性别:");
String gender = scanner.nextLine();
System.out.print("职位:");
String position = scanner.nextLine();
System.out.print("年龄:");
int age = scanner.nextInt();
System.out.print("月薪:");
double salary = scanner.nextDouble();
System.out.print("部门编号:");
int departmentId = scanner.nextInt();
Employee employee = new Employee(id, name, gender, position, age, salary, departmentId);
system.addEmployee(employee);
break;
case 2:
system.displayAllEmployees();
break;
case 3:
System.out.print("请输入要查询的员工工号:");
int displayId = scanner.nextInt();
system.displayEmployeeById(displayId);
break;
case 4:
System.out.print("请输入要修改薪资的员工工号:");
int modifyId = scanner.nextInt();
System.out.print("请输入新的薪资:");
double newSalary = scanner.nextDouble();
system.modifyEmployeeSalary(modifyId, newSalary);
break;
case 5:
System.out.print("请输入要查询的部门编号:");
int departmentIdToDisplay = scanner.nextInt();
system.displayEmployeesByDepartmentId(departmentIdToDisplay);
break;
case 0:
System.out.println("程序已退出。");
return;
default:
System.out.println("无效的选择!");
break;
}
}
}
}
```
这是一个简单的企业人事管理系统,你可以根据自己的需求进行修改和扩展。希望对你有帮助!如果还有其他问题,请随时提问。
相关推荐
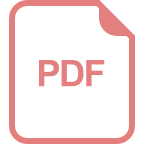














