java中StringRedisTemplate实现排行榜的代码
时间: 2024-10-26 13:04:13 浏览: 10
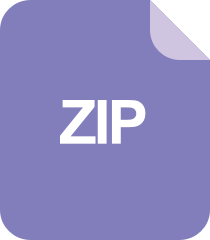
基于Java语言的Redis Zset实现奥运金牌排行榜设计源码
在Java中,使用`StringRedisTemplate`实现排行榜功能通常涉及到对Redis的数据结构,如有序集合(Sorted Set)的熟练应用。这里是一个简单的示例,展示如何使用`StringRedisTemplate`操作Redis来存储和获取排行榜:
```java
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ZSetOperations;
public class RedisRankingService {
private final StringRedisTemplate stringRedisTemplate;
private final String rankingKey; // 排行榜的键
public RedisRankingService(StringRedisTemplate stringRedisTemplate, String rankingKey) {
this.stringRedisTemplate = stringRedisTemplate;
this.rankingKey = rankingKey;
}
/**
* 添加用户到排行榜
*/
public void addUserToRanking(String userId, int score) {
ZSetOperations<String, Integer> zsetOps = stringRedisTemplate.opsForZSet();
zsetOps.add(rankingKey, userId, score);
}
/**
* 获取排行榜前n名
*/
public List<String> getTopNUsers(int n) {
ZSetOperations<String, Double> ops = stringRedisTemplate.opsForZSet();
return ops.zRangeByScore(rankingKey, 0.0, Double.MAX_VALUE, true, n);
}
/**
* 更新用户的分数并调整排行榜
*/
public void updateUserScore(String userId, int newScore) {
ZSetOperations<String, Integer> zsetOps = stringRedisTemplate.opsForZSet();
zsetOps.withSCORES().move(userId, newScore);
}
// 其他辅助方法...
}
```
在这个例子中,我们创建了一个名为`RedisRankingService`的服务,它包含了添加用户、获取排行榜和更新用户分数的方法。`addUserToRanking`用于将用户ID及其得分添加到有序集合中,`getTopNUsers`则从集合中取出得分最高的前n名用户,`updateUserScore`用于更新指定用户分数并保持排行榜排序。
阅读全文
相关推荐
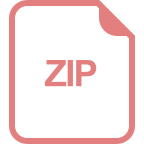
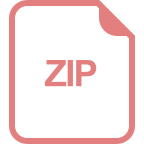
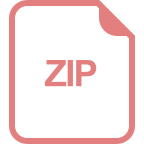
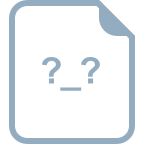
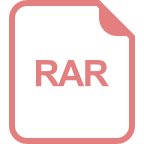
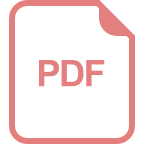
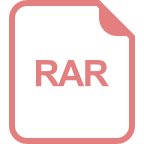
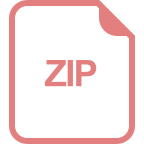
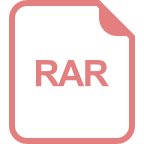
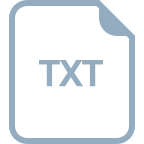
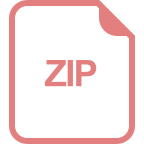