C#写一段代码功能为在指定文件夹检索某一文件
时间: 2024-09-10 17:16:21 浏览: 82
在C#中,你可以使用`Directory.GetFiles`方法从特定目录检索文件。下面是一个简单的示例,它会搜索指定文件夹及其子文件夹,查找具有特定名称的文件:
```csharp
using System.IO;
public List<string> FindFilesInFolder(string folderPath, string fileNamePattern)
{
return Directory.GetFiles(folderPath, fileNamePattern, SearchOption.AllDirectories).ToList();
}
// 调用函数
List<string> matchingFiles = FindFilesInFolder(@"C:\YourFolderPath", "*.txt"); // 搜索名为".txt"的所有文本文件
```
在这个例子中,`folderPath`是你想要搜索的文件夹路径,`fileNamePattern`是你希望匹配的文件名模式(如`.txt`表示所有.txt文件)。`SearchOption.AllDirectories`告诉`GetFiles`方法搜索整个树形结构。
相关问题
C#写一段代码功能为在指定文件夹检索指定名称文件
在C#中,你可以使用`Directory.GetFiles()`函数来搜索指定文件夹中特定名称的文件。以下是一个简单的示例代码:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
// 定义要搜索的文件夹路径和文件名
string folderPath = @"C:\YourFolderPath\";
string fileNamePattern = "yourFileNamePattern.txt";
// 获取文件列表
string[] files = Directory.GetFiles(folderPath, fileNamePattern, SearchOption.AllDirectories);
if (files.Length > 0)
{
Console.WriteLine($"找到以下匹配的文件:");
foreach (string file in files)
{
Console.WriteLine(file);
}
}
else
{
Console.WriteLine("未找到匹配的文件。");
}
// 程序结束
Console.ReadLine();
}
}
```
在这个代码中,你需要将`"C:\YourFolderPath\"`替换为你实际想要搜索的文件夹路径,`"yourFileNamePattern.txt"`替换为你需要查找的文件名模式。`SearchOption.AllDirectories`参数使得搜索可以递归地遍历所有子目录。
c# 检索指定文件夹下所有字符串,获取"sfun.UpOperation"和“global”之间的字符串
你可以使用 C# 中的 `Directory` 和 `File` 类来遍历指定文件夹下的所有文件,然后使用 `StreamReader` 来读取文件内容。对于每个文件的内容,你可以使用正则表达式来查找指定的字符串。以下是一个示例代码:
```csharp
using System.IO;
using System.Text.RegularExpressions;
string folderPath = "your/folder/path";
string pattern = @"sfun\.UpOperation\(""([^""]*)""(?:(?!global).)*";
foreach (string filePath in Directory.GetFiles(folderPath))
{
using (StreamReader reader = new StreamReader(filePath))
{
string content = reader.ReadToEnd();
MatchCollection matches = Regex.Matches(content, pattern);
foreach (Match match in matches)
{
string value = match.Groups[1].Value;
Console.WriteLine(value);
}
}
}
```
这段代码会遍历指定文件夹下的所有文件,读取每个文件的内容,并使用正则表达式 `@"sfun\.UpOperation\(""([^""]*)""(?:(?!global).)*"` 查找所有匹配的字符串。其中,`([^"]*)` 表示匹配零个或多个非双引号字符,这样就可以获取在 `sfun.UpOperation(` 和 `)` 之间的字符串。`(?:(?!global).)*` 表示匹配任意数量的字符,但不包含 `global` 字符串。这样就可以确保匹配的字符串不包含 `global`。
阅读全文
相关推荐
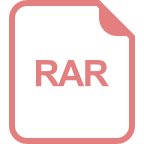
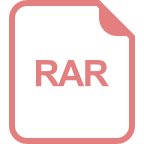
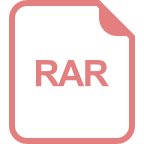
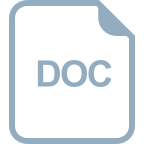
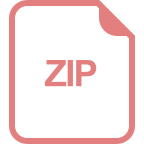
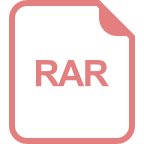
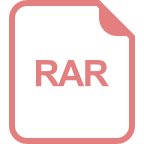
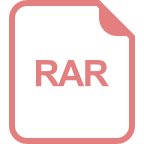
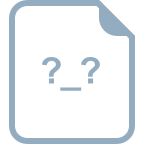
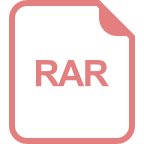
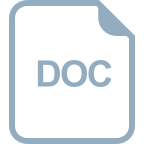
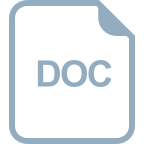
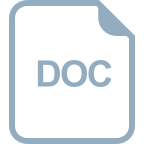
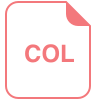
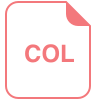

