实现一个购物车应用程序,可以在采购网页进行购物、显示目前采购项目数量,并可查看购物车内容。使用jdk8,idea,Maven,等工具生成代码
时间: 2024-12-20 16:23:03 浏览: 11
为了实现一个简单的购物车应用,我们将创建一个Java项目,使用Maven作为构建工具,并利用JDK 8的新特性。这里是一个基本的框架代码示例:
首先,你需要设置一个`Cart`类,用于存储商品及其数量:
```java
import java.util.HashMap;
import java.util.Map;
public class Cart {
private Map<String, Integer> items = new HashMap<>();
public void addItem(String product, int quantity) {
if (items.containsKey(product)) {
items.put(product, items.get(product) + quantity);
} else {
items.put(product, quantity);
}
}
public void removeItem(String product, int quantity) {
if (items.containsKey(product)) {
int currentQuantity = items.get(product);
if (currentQuantity >= quantity) {
items.put(product, currentQuantity - quantity);
} else {
throw new IllegalArgumentException("Insufficient quantity for " + product);
}
}
}
public int getTotalItems() {
return items.values().stream().mapToInt(Integer::intValue).sum();
}
public String getContents() {
StringBuilder cartContent = new StringBuilder();
for (Map.Entry<String, Integer> entry : items.entrySet()) {
cartContent.append(entry.getKey()).append(": ").append(entry.getValue()).append(", ");
}
// Remove last ", " character
cartContent.setLength(cartContent.length() - 2);
return cartContent.toString();
}
}
```
然后,你可以创建一个`ShoppingPage`类,包含用户交互功能:
```java
import javax.swing.*;
import java.awt.*;
public class ShoppingPage extends JFrame {
private JLabel itemCountLabel;
private JButton addToCartButton;
private JButton viewCartButton;
public ShoppingPage(Cart cart) {
// Initialize components and layout...
itemCountLabel = new JLabel("0 items in cart");
addToCartButton.addActionListener(e -> cart.addItem("Product", 1));
viewCartButton.addActionListener(e -> JOptionPane.showMessageDialog(this, cart.getContents()));
// ...add components to the frame and handle events...
}
}
// Main method or a Controller class can be created like this:
public static void main(String[] args) {
Cart cart = new Cart();
ShoppingPage shoppingPage = new ShoppingPage(cart);
shoppingPage.setSize(400, 300);
shoppingPage.setVisible(true);
}
```
这个例子非常基础,实际应用可能会更复杂,包括数据库操作、持久化、错误处理以及前端界面设计。如果你想了解更多关于如何将这个结构扩展到Maven项目并集成到Idea IDE中,可以考虑创建一个pom.xml文件,配置Spring Boot MVC或者直接使用Swing进行GUI设计。
阅读全文
相关推荐
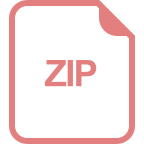
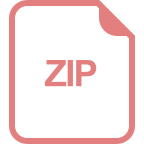
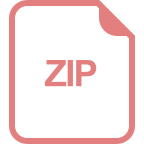
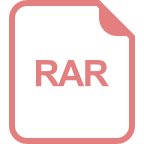
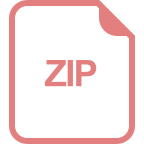
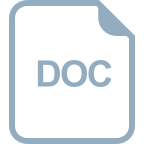
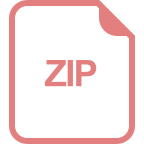
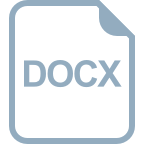
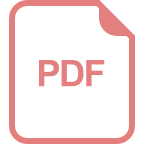
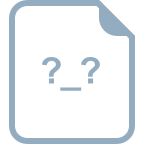
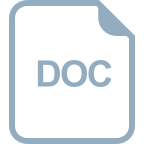
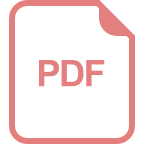
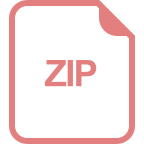
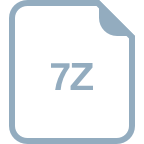
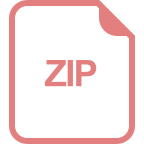
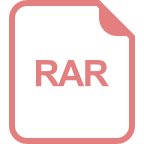
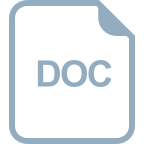
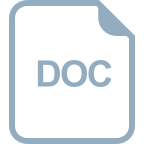
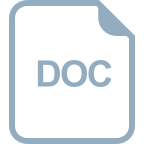