给你一个链表的头节点 head 和一个整数 val ,请你删除链表中所有满足 Node.val == val 的节点,并返回 新的头节点 输入:head = [1,2,6,3,4,5,6], val = 6
时间: 2024-10-06 13:05:45 浏览: 15
要删除链表中所有值为 `val` 的节点,你可以遍历链表,对于每个节点,如果它的值等于 `val`,就跳过当前节点并继续向下遍历;否则,将其指向下个节点。这里有一个简单的 C 语言算法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
// 创建新节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 删除链表中的重复值
ListNode* removeDuplicates(ListNode* head, int val) {
if (head == NULL || head->val != val)
return head; // 如果链表为空或头节点不是目标值,直接返回
ListNode* curr = head;
while (curr != NULL && curr->next != NULL) { // 遍历到链表末尾
if (curr->next->val == val) { // 找到目标值
ListNode* temp = curr->next; // 保存下一个节点
curr->next = curr->next->next; // 删除当前节点
free(temp); // 释放已删除节点的内存
} else {
curr = curr->next;
}
}
return head;
}
// 打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->val);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
ListNode* head = createNode(1);
head->next = createNode(2);
head->next->next = createNode(6);
head->next->next->next = createNode(3);
head->next->next->next->next = createNode(4);
head->next->next->next->next->next = createNode(5);
head->next->next->next->next->next = createNode(6);
int val = 6;
head = removeDuplicates(head, val);
printf("New linked list after removing duplicates of %d:\n", val);
printList(head);
return 0;
}
```
这段代码首先定义了一个链表节点结构体 `ListNode`,然后实现了创建新节点、删除重复值以及打印链表的功能。在 `main()` 函数中,创建了一个包含重复值 6 的链表,调用 `removeDuplicates()` 函数后,打印出新的链表。
阅读全文
相关推荐
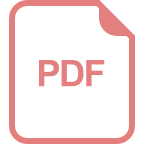
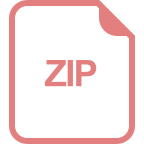
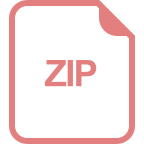















