qt 触摸屏双指放缩qgraphicsitem
时间: 2024-02-04 21:10:02 浏览: 410
在Qt中,可以使用QGraphicsItem类来创建可交互的图形项。要实现触摸屏双指放缩功能,可以按照以下步骤进行操作:
1. 创建一个自定义的QGraphicsItem子类,例如MyGraphicsItem,用于显示和处理图形项的内容。
2. 在MyGraphicsItem类中,重写mousePressEvent、mouseMoveEvent和wheelEvent等事件处理函数,以便捕获触摸屏的手势操作。
3. 在mousePressEvent事件中,记录下触摸屏上第一个手指的位置。
4. 在mouseMoveEvent事件中,计算两个手指之间的距离变化,并根据变化的比例来调整图形项的大小。
5. 在wheelEvent事件中,根据滚轮的滚动方向来调整图形项的大小。
下面是一个简单的示例代码,演示了如何实现触摸屏双指放缩功能:
```cpp
#include <QGraphicsItem>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QTouchEvent>
class MyGraphicsItem : public QGraphicsItem
{
public:
MyGraphicsItem()
{
setFlag(ItemIsMovable);
}
QRectF boundingRect() const override
{
return QRectF(0, 0, 100, 100);
}
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override
{
painter->drawRect(boundingRect());
}
void mousePressEvent(QGraphicsSceneMouseEvent *event) override
{
if (event->buttons() & Qt::LeftButton) {
// 记录第一个手指的位置
m_startPos = event->scenePos();
}
QGraphicsItem::mousePressEvent(event);
}
void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override
{
if (event->buttons() & Qt::LeftButton) {
// 计算两个手指之间的距离变化
QPointF newPos = event->scenePos();
qreal distance = QLineF(m_startPos, newPos).length();
// 根据距离变化来调整图形项的大小
qreal scaleFactor = distance / 100.0;
setScale(scaleFactor);
}
QGraphicsItem::mouseMoveEvent(event);
}
void wheelEvent(QGraphicsSceneWheelEvent *event) override
{
// 根据滚轮的滚动方向来调整图形项的大小
qreal scaleFactor = event->delta() > 0 ? 1.1 : 0.9;
setScale(scaleFactor);
event->accept();
}
private:
QPointF m_startPos;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QGraphicsScene scene;
MyGraphicsItem item;
scene.addItem(&item);
QGraphicsView view(&scene);
view.setRenderHint(QPainter::Antialiasing);
view.show();
return app.exec();
}
```
这个示例代码创建了一个自定义的图形项MyGraphicsItem,并将其添加到QGraphicsScene中。在MyGraphicsItem中,重写了mousePressEvent、mouseMoveEvent和wheelEvent等事件处理函数,实现了触摸屏双指放缩的功能。
希望这个示例能够帮助到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐

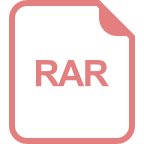












