Vue3中pinia中在state中定义的数据getters中使用this获取不到
时间: 2024-09-15 19:07:24 浏览: 90
在Vue3中,Pinia是一个状态管理库,它基于Vue3的Composition API设计。当你在`store`的`state`或` getters`中定义数据时,`this`关键字通常用于引用当前组件实例,但在`getters`中直接使用`this`可能会出现问题。
这是因为`getters`会被视为普通的计算属性,它们会在每次依赖改变时重新计算。由于`getters`不会绑定到任何特定的组件实例上,所以在`getters`内部直接使用`this`会得到`undefined`,因为`this`并不是指向组件实例。
解决这个问题的方法是在`getters`中显式地传入`store`实例或者存储状态的变量,然后通过那个实例来访问状态。例如:
```javascript
import { defineStore } from 'pinia';
const store = defineStore('myStore', {
state() {
return {
count: 0,
}
},
getters: {
getCount(state) {
return state.count;
},
},
});
// 使用时,在组件里这样做
export default {
computed: {
countFromGetter() {
return this.$store.getters.getCount; // 这样就能访问到count
}
},
};
```
相关问题
vue3 使用pinia数据存储
Vue 3 的 Pinia 是一个基于 Composition API 的状态管理库,用于在 Vue 3 应用程序中管理数据。下面是使用 Pinia 进行数据存储的一般步骤:
1. 安装 Pinia:
```bash
npm install pinia
```
2. 创建一个 Pinia 实例并导出它,以便在应用程序的入口文件中使用:
```javascript
// src/store/index.js
import { createPinia } from 'pinia';
export const pinia = createPinia();
```
3. 在应用程序入口文件(例如 `main.js`)中使用创建的 Pinia 实例:
```javascript
import { createApp } from 'vue';
import App from './App.vue';
import { pinia } from './store';
const app = createApp(App);
app.use(pinia);
app.mount('#app');
```
4. 创建一个 store 文件,定义数据存储和相关操作:
```javascript
// src/store/count.js
import { defineStore } from 'pinia';
export const useCountStore = defineStore('count', {
state: () => ({
count: 0,
}),
getters: {
doubleCount() {
return this.count * 2;
},
},
actions: {
increment() {
this.count++;
},
decrement() {
this.count--;
},
},
});
```
5. 在组件中使用数据存储:
```vue
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="decrement">Decrement</button>
</div>
</template>
<script>
import { useCountStore } from '../store/count';
export default {
setup() {
const countStore = useCountStore();
return {
count: countStore.count,
doubleCount: countStore.doubleCount,
increment: countStore.increment,
decrement: countStore.decrement,
};
},
};
</script>
```
通过以上步骤,你可以在 Vue 3 应用程序中使用 Pinia 进行数据存储。你可以定义状态、计算属性和操作,并在组件中使用它们。
vue3使用pinia使用
Pinia 是 Vue.js 的状态管理库,它在 Vue 3 中得到了原生支持。Pinia 是基于 Composition API 设计的,相比于 Vue 2 的 Vuex,Pinia 更简洁、更容易使用,并且拥有了更好的 TypeScript 支持。
要使用 Pinia,首先需要安装它:
```bash
npm install pinia
```
然后,在你的 Vue 3 应用中引入并使用 Pinia:
```javascript
import { createApp } from 'vue'
import { createPinia } from 'pinia'
import App from './App.vue'
const app = createApp(App)
// 创建 Pinia 实例
const pinia = createPinia()
// 将 Pinia 实例添加到 Vue 应用中
app.use(pinia)
app.mount('#app')
```
接下来,你可以定义你的 store。一个基本的 Pinia store 包含了状态、getters、actions 和选项:
```javascript
// src/stores/counter.js
import { defineStore } from 'pinia'
export const useCounterStore = defineStore('counter', {
state: () => {
return { count: 0 }
},
getters: {
doubleCount: (state) => state.count * 2,
},
actions: {
increment() {
this.count++
},
},
})
```
在组件中使用 store 的步骤如下:
```vue
<template>
<div>
<p>Count is: {{ counterStore.count }}</p>
<p>Double count is: {{ counterStore.doubleCount }}</p>
<button @click="counterStore.increment">Increment</button>
</div>
</template>
<script>
import { defineComponent } from 'vue'
import { useCounterStore } from '../stores/counter'
export default defineComponent({
setup() {
const counterStore = useCounterStore()
return {
counterStore,
}
},
})
</script>
```
通过以上步骤,你可以在 Vue 3 应用中成功地使用 Pinia 进行状态管理。
阅读全文
相关推荐
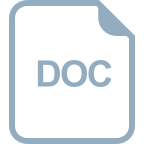
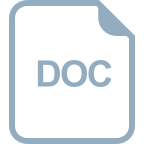
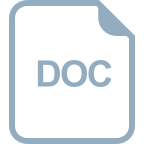
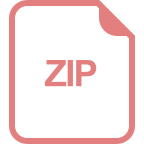
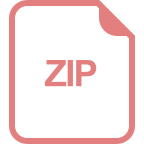











