java 下载文件到本地
时间: 2023-09-25 10:14:19 浏览: 158
在 Java 中,你可以使用 Java 的标准库来下载文件到本地。你可以使用 `java.net.URL` 和 `java.nio.file` 类来实现这个功能。下面是一个简单的示例代码:
```java
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class FileDownloader {
public static void main(String[] args) {
String fileUrl = "https://example.com/file.txt";
String savePath = "C:/path/to/save/file.txt";
try {
// 方法一:使用 InputStream 和 FileOutputStream
downloadFileUsingStream(fileUrl, savePath);
// 方法二:使用 Files.copy
downloadFileUsingFilesCopy(fileUrl, savePath);
System.out.println("文件下载成功!");
} catch (IOException e) {
System.out.println("文件下载失败: " + e.getMessage());
}
}
private static void downloadFileUsingStream(String fileUrl, String savePath) throws IOException {
URL url = new URL(fileUrl);
try (BufferedInputStream in = new BufferedInputStream(url.openStream());
FileOutputStream fileOutputStream = new FileOutputStream(savePath)) {
byte[] dataBuffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(dataBuffer, 0, 1024)) != -1) {
fileOutputStream.write(dataBuffer, 0, bytesRead);
}
}
}
private static void downloadFileUsingFilesCopy(String fileUrl, String savePath) throws IOException {
URL url = new URL(fileUrl);
Path targetPath = Path.of(savePath);
Files.copy(url.openStream(), targetPath, StandardCopyOption.REPLACE_EXISTING);
}
}
```
你需要将 `fileUrl` 替换为要下载的文件的 URL,将 `savePath` 替换为要保存到的本地文件路径。这个示例代码提供了两种下载文件的方法,你可以选择其中一种来使用。
阅读全文
相关推荐
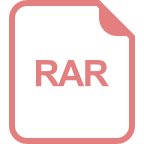
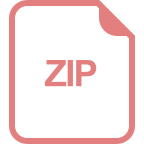
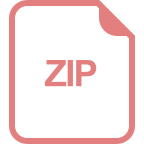
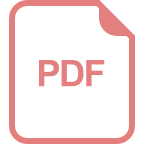
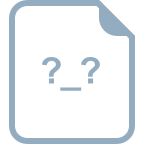
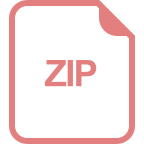
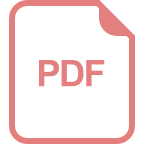
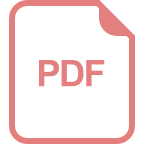
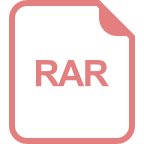
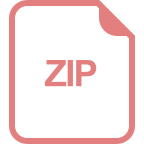
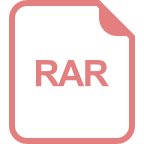
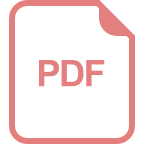