编写三层架构,使用注解管理bean并实现自动装配,在测试类中获取bean,成功调用controller层的方法。
时间: 2024-10-15 22:15:42 浏览: 44
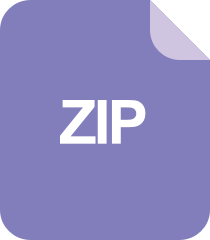
SpringBoot之Bean的使用及处理
在Java中,三层架构通常指的是控制器(Controller)、服务层(Service)和持久层(Repository)。在Spring框架下,可以使用注解轻松地管理和组织Bean,并实现自动装配(Inversion of Control, IoC)。这里是一个简化版的步骤说明:
1. **创建@Component 注解的类**:
- 在Spring MVC项目中,`@Component`用于标记需要管理的Bean。例如,对于Service和Repository层:
```java
@Service
public class YourService {
// Service方法...
}
@Repository
public interface YourRepository extends JpaRepository<Book, Long> {
// Repository方法...
}
```
2. **@Autowired 自动装配**:
- 在Controller层或其它需要依赖的类上,使用`@Autowired`注解自动注入Service和Repository层的Bean。例如:
```java
@RestController
public class YourController {
@Autowired
private YourService yourService;
@GetMapping("/your-endpoint")
public ResponseEntity<?> processRequest() {
// 调用Service方法
Object result = yourService.yourMethod();
// 返回响应...
}
}
```
3. **编写测试类**:
- 使用Spring Test框架,如`@RunWith(SpringRunner.class)`和`@ContextConfiguration`注解来加载Spring容器,然后在测试类中注入需要的Bean来测试Controller方法。例如:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class YourControllerTest {
@Autowired
private YourController yourController;
@Autowired
private YourService yourService; // 如果你想直接测试Service
@Test
public void testProcessRequest() {
// 在测试方法里调用Controller的方法,检查返回结果
Object result = yourController.processRequest();
// 测试断言...
}
}
```
在测试类中,Spring会根据@Autowired注解找到相应的Bean进行实例化,并注入到需要的地方。
阅读全文
相关推荐
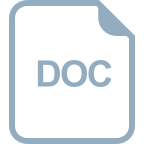
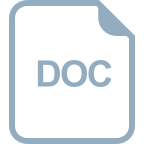
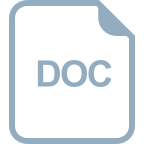
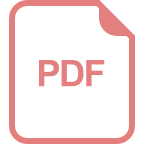
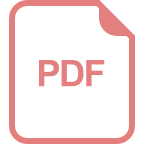
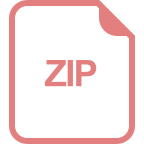
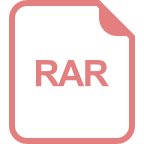
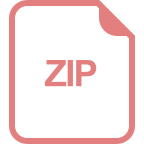
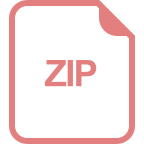
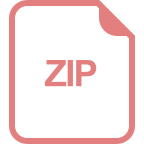
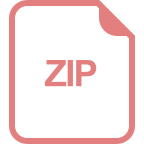
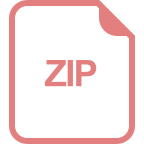
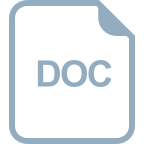
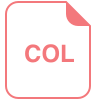
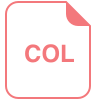
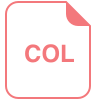
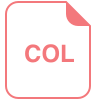
