matplotlib 多图排版
时间: 2023-09-14 11:14:52 浏览: 88
对于matplotlib多图排版,你可以使用subplot,gridspec或subplots来实现。
1. 使用subplot函数:
subplot函数允许您在一个Figure对象中创建多个子图。它接受三个参数:行数、列数和子图索引。
以下是一个简单的例子,展示如何在一个Figure中创建2x2的子图布局:
```python
import matplotlib.pyplot as plt
# 创建Figure对象,并设置子图布局为2行2列
fig = plt.figure()
# 创建子图1,并设为第1个位置
ax1 = fig.add_subplot(2, 2, 1)
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3])
# 创建子图2,并设为第2个位置
ax2 = fig.add_subplot(2, 2, 2)
ax2.plot([1, 2, 3, 4], [4, 2, 3, 1])
# 创建子图3,并设为第3个位置
ax3 = fig.add_subplot(2, 2, 3)
ax3.plot([1, 2, 3, 4], [3, 1, 4, 2])
# 创建子图4,并设为第4个位置
ax4 = fig.add_subplot(2, 2, 4)
ax4.plot([1, 2, 3, 4], [2, 3, 1, 4])
# 显示图形
plt.show()
```
2. 使用gridspec模块:
gridspec模块提供了更灵活的子图布局选项。您可以使用gridspec.GridSpec将Figure分割成不规则的网格,并在每个网格中放置子图。
以下是一个示例代码,展示如何使用gridspec实现2x2的子图布局:
```python
import matplotlib.pyplot as plt
from matplotlib import gridspec
# 创建Figure对象
fig = plt.figure()
# 使用gridspec将Figure分割成2行2列
gs = gridspec.GridSpec(2, 2)
# 在第1行第1列创建子图
ax1 = fig.add_subplot(gs[0, 0])
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3])
# 在第1行第2列创建子图
ax2 = fig.add_subplot(gs[0, 1])
ax2.plot([1, 2, 3, 4], [4, 2, 3, 1])
# 在第2行第1列创建子图
ax3 = fig.add_subplot(gs[1, 0])
ax3.plot([1, 2, 3, 4], [3, 1, 4, 2])
# 在第2行第2列创建子图
ax4 = fig.add_subplot(gs[1, 1])
ax4.plot([1, 2, 3, 4], [2, 3, 1, 4])
# 显示图形
plt.show()
```
3. 使用subplots函数:
subplots函数可以同时创建多个子图,并返回一个包含所有子图的Figure对象和Axes对象的数组。
以下是一个示例代码,展示如何使用subplots函数创建2x2的子图布局:
```python
import matplotlib.pyplot as plt
# 使用subplots函数创建2x2的子图布局
fig, axs = plt.subplots(2, 2)
# 在第1行第1列创建子图
axs[0, 0].plot([1, 2, 3, 4], [1, 4, 2, 3])
# 在第1行第2列创建子图
axs[0, 1].plot([1, 2, 3, 4], [4, 2, 3, 1])
# 在第2行第1列创建子图
axs[1, 0].plot([1, 2, 3, 4], [3, 1, 4, 2])
# 在第2行第2列创建子图
axs[1, 1].plot([1, 2, 3, 4], [2, 3, 1, 4])
# 调整子图之间的间距
plt.tight_layout()
# 显示图形
plt.show()
```
这些是matplotlib中几种常见的多图排版方法。您可以根据需要选择适合您的情况的方法。
阅读全文
相关推荐
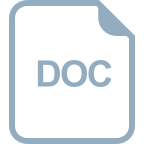
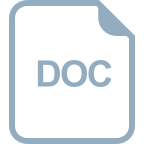
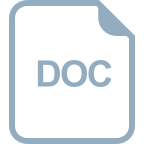
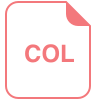
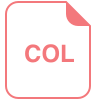

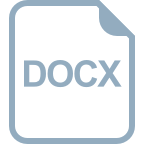
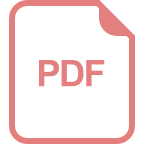
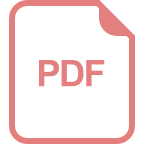
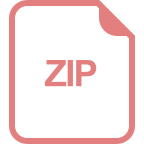
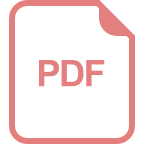
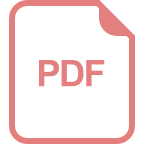
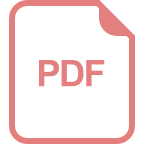
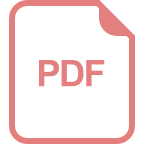
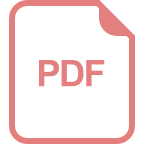
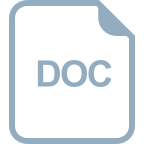
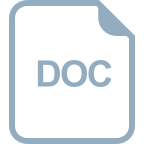
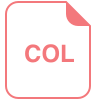
