vue 字典动态添加键
时间: 2023-10-03 08:04:56 浏览: 91
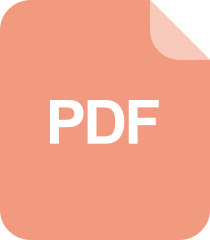
详解Vue 动态添加模板的几种方法
在Vue中,要动态添加字典的键,可以使用以下方法:
1. 使用`Vue.set()`方法:`Vue.set(target, key, value)`。其中,`target`是要添加键的目标对象,`key`是要添加的键,`value`是要设置的值。
例如:
```javascript
Vue.set(this.dictionary, 'newKey', 'newValue');
```
2. 使用`this.$set()`方法:`this.$set(target, key, value)`。与`Vue.set()`方法类似,只是通过Vue实例的属性来调用。
例如:
```javascript
this.$set(this.dictionary, 'newKey', 'newValue');
```
这两种方法都会触发Vue的响应式系统,确保动态添加的键也能被Vue追踪和更新。
阅读全文
相关推荐
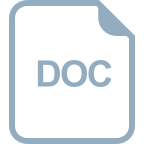
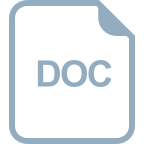
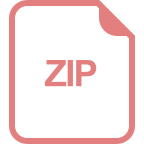
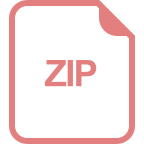
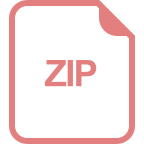
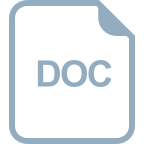
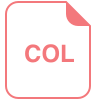
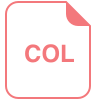
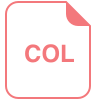




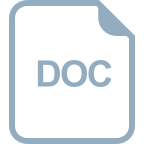
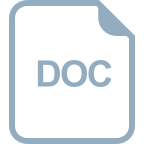
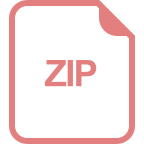