python线性回归算法代码
时间: 2023-12-06 10:38:37 浏览: 35
以下是Python实现一元线性回归的代码,包括假设函数、损失函数和梯度下降法:
```python
import numpy as np
# 定义假设函数
def hypothesis(theta, X):
return np.dot(X, theta)
# 定义损失函数
def cost_function(theta, X, y):
m = len(y)
J = np.sum((hypothesis(theta, X) - y) ** 2) / (2 * m)
return J
# 定义梯度下降函数
def gradient_descent(theta, X, y, alpha, num_iters):
m = len(y)
J_history = np.zeros(num_iters)
for i in range(num_iters):
theta = theta - alpha / m * np.dot(X.T, (hypothesis(theta, X) - y))
J_history[i] = cost_function(theta, X, y)
return theta, J_history
```
其中,假设函数hypothesis(theta, X)用于计算预测值,损失函数cost_function(theta, X, y)用于计算预测值与真实值之间的误差,梯度下降函数gradient_descent(theta, X, y, alpha, num_iters)用于更新参数theta,使得损失函数最小化。
下面是一个使用上述代码进行一元线性回归的例子:
```python
import matplotlib.pyplot as plt
# 构造数据
X = np.array([1, 2, 3, 4, 5])
y = np.array([3, 5, 7, 9, 11])
# 在X前面添加一列1,用于计算截距
X = np.vstack([np.ones(len(X)), X]).T
# 初始化参数
theta = np.zeros(2)
# 设置学习率和迭代次数
alpha = 0.01
num_iters = 1000
# 进行梯度下降
theta, J_history = gradient_descent(theta, X, y, alpha, num_iters)
# 输出最终的参数和损失函数值
print('theta:', theta)
print('cost:', cost_function(theta, X, y))
# 绘制数据和拟合直线
plt.scatter(X[:, 1], y)
plt.plot(X[:, 1], hypothesis(theta, X))
plt.show()
```
相关推荐
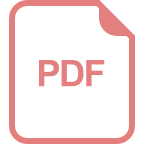
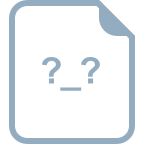














