java实现word文档转换成html格式文本
时间: 2023-09-15 10:19:48 浏览: 232
您可以使用Apache POI库来实现将Word文档转换为HTML格式文本。以下是一个简单的Java代码示例:
```java
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.converter.WordToHtmlConverter;
import org.apache.poi.hwpf.usermodel.Range;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import java.io.*;
public class WordToHtmlConverterExample {
public static void main(String[] args) {
String inputFilePath = "input.doc";
String outputFilePath = "output.html";
try (InputStream inputStream = new FileInputStream(inputFilePath);
HWPFDocument document = new HWPFDocument(new POIFSFileSystem(inputStream));
Writer writer = new BufferedWriter(new FileWriter(outputFilePath))) {
Range range = document.getRange();
WordToHtmlConverter converter = new WordToHtmlConverter(DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument());
converter.processDocument(document);
org.w3c.dom.Document htmlDocument = converter.getDocument();
writer.write(htmlDocumentToString(htmlDocument));
} catch (IOException | ParserConfigurationException e) {
e.printStackTrace();
}
}
private static String htmlDocumentToString(org.w3c.dom.Document document) throws IOException {
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
StringWriter writer = new StringWriter();
transformer.transform(new DOMSource(document), new StreamResult(writer));
return writer.toString();
}
}
```
请确保在运行代码之前已经将Apache POI库添加到您的项目中。此代码将读取名为`input.doc`的Word文档,并将其转换为HTML格式,并将结果写入名为`output.html`的文件中。
请注意,这只是一个简单的示例,对于更复杂的文档,可能需要额外的处理和调整来保留样式和格式。
阅读全文
相关推荐
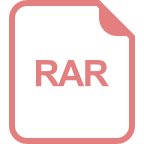
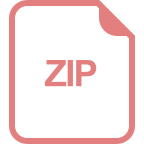
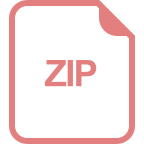
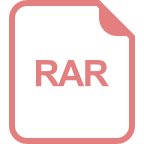






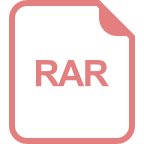
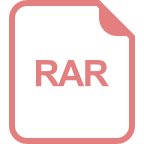
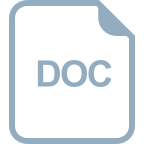
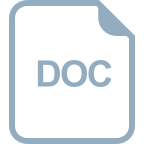
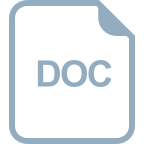

