完善以上代码,使其更加完善
时间: 2024-10-05 09:01:54 浏览: 27
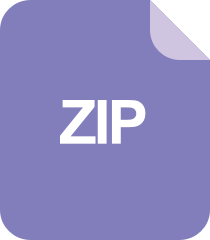
js代码-封装完善自己的JS代码库
为了完善上述代码,我们可以做以下几个方面的改进:
1. 错误检查:添加输入验证,确保用户提供的矩阵尺寸和幂次有效,并检查内存分配是否成功。
2. 稀疏矩阵支持:如果矩阵大部分元素为零,可以考虑使用稀疏矩阵数据结构,减少内存消耗。
3. 更好的性能优化:对于大矩阵,可以采用分块矩阵乘法(Strassen's Algorithm 或者 CUDA 并行化)提升效率。
4. 输入和输出格式:提供更友好的用户界面,例如让用户可以选择输入矩阵的方式,或者输出结果时按行或列显示。
下面是针对上述改进的完善版本:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 使用头文件实现大整数操作(如 GMP)
#include "mpmath.h"
// 稀疏矩阵结构体
typedef struct SparseMatrix {
long row[2], col[2], val[2]; // 一个二元组表示一个非零元素
} SparseMatrix;
// 转换密集到稀疏矩阵
SparseMatrix dense_to_sparse(int **matrix, int n) {
SparseMatrix sparse;
int count = 0;
for (int i = 0; i < n; ++i) {
for (int j = 0; j < n; ++j) {
if (matrix[i][j]) {
sparse.row[count] = i;
sparse.col[count] = j;
sparse.val[count++] = matrix[i][j];
}
}
}
return sparse;
}
// 计算幂并返回稀疏矩阵
SparseMatrix sparse_matrix_pow(SparseMatrix a, long k) {
SparseMatrix res;
while (k > 0) {
if (k % 2 == 1) {
res = sparse_matrix_multiply(res, a);
}
a = sparse_matrix_multiply(a, a);
k /= 2;
}
return res;
}
// 矩阵乘法函数 (仅适用于稀疏矩阵)
SparseMatrix sparse_matrix_multiply(SparseMatrix a, SparseMatrix b) {
// ... 实现稀疏矩阵乘法 ...
}
int main() {
int n, k;
printf("Enter the size of the square matrix and its power: ");
scanf("%d %ld", &n, &k);
if (n <= 0 || k < 0) {
printf("Invalid input. Please enter positive integers.\n");
return 1;
}
int **dense = malloc(n * sizeof(int *));
for (int i = 0; i < n; ++i) {
dense[i] = malloc(n * sizeof(int));
}
// 用户输入密集矩阵,转换为稀疏形式
printf("Enter the elements of the dense matrix:\n");
for (int i = 0; i < n; ++i) {
for (int j = 0; j < n; ++j) {
scanf("%d", dense[i]+j);
}
}
SparseMatrix input = dense_to_sparse(dense, n);
// 计算幂并输出结果
SparseMatrix result = sparse_matrix_pow(input, k);
printf("Resultant sparse matrix after powering:\n");
// 输出稀疏矩阵,根据需要自定义格式
// 清理内存
for (int i = 0; i < n; ++i) {
free(dense[i]);
}
free(dense);
return 0;
}
```
在这个版本中,我们引入了稀疏矩阵的概念,这有助于处理大量稀疏数据。注意,`sparse_matrix_multiply` 和 `sparse_matrix_pow` 需要单独实现稀疏矩阵的乘法运算,这部分未在上面展示,因为它们的具体实现取决于使用的数据结构和算法。
阅读全文
相关推荐
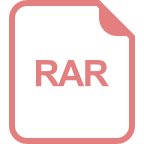
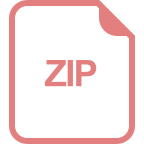
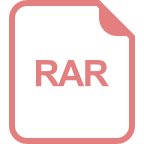
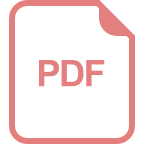
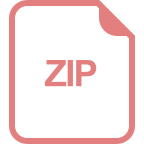
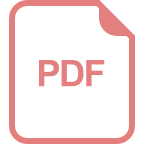
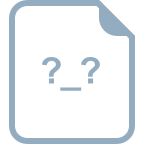
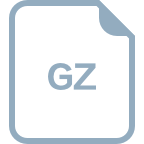
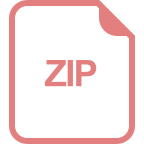
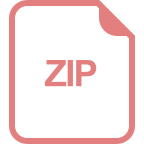
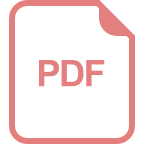
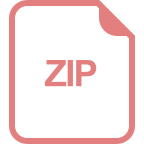