16进制转为二进制 c++
时间: 2024-10-27 19:04:31 浏览: 8
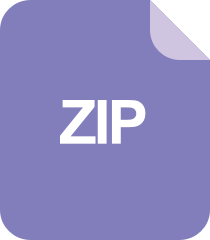
顺序栈、链栈将10进制转为2、8、16进制源码

在C++中,将十六进制数转换成二进制数可以使用内置的`bitset`库或者手动转换字符串。下面是两种常见的方法:
1. 使用`bitset`库:
```cpp
#include <bitset>
#include <string>
std::string hex_to_binary(std::string hex_num) {
std::bitset<32> binary; // 假设最多16位的十六进制,32位可以表示
for (size_t i = 0; i < hex_num.length(); i++) {
if (hex_num[i] >= '0' && hex_num[i] <= '9') { // A-F之间的字符代表10-15
binary.set(i * 4, static_cast<int>(hex_num[i] - '0')); // 高四位一组
} else if (hex_num[i] >= 'A' && hex_num[i] <= 'F') {
binary.set(i * 4, static_cast<int>(hex_num[i] - 'A' + 10)); // 转换大写字母到数字
} else if (hex_num[i] >= 'a' && hex_num[i] <= 'f') {
binary.set(i * 4, static_cast<int>(hex_num[i] - 'a' + 10)); // 小写字母同样处理
} else {
throw std::invalid_argument("Invalid hexadecimal character");
}
}
return binary.to_string();
}
// 示例用法
int main() {
std::string hex = "FF"; // 十六进制数
std::cout << "Binary representation of " << hex << ": " << hex_to_binary(hex) << std::endl;
return 0;
}
```
2. 手动转换字符串:
```cpp
#include <string>
std::string hex_to_binary(const std::string& hex_num) {
std::string binary = "";
for (char ch : hex_num) {
int dec_value;
if (ch >= '0' && ch <= '9')
dec_value = ch - '0';
else if (ch >= 'A' && ch <= 'F')
dec_value = ch - 'A' + 10;
else if (ch >= 'a' && ch <= 'f')
dec_value = ch - 'a' + 10;
else
throw std::invalid_argument("Invalid hexadecimal character");
binary += std::to_string(dec_value * 16) + binary;
}
return binary;
}
```
阅读全文
相关推荐
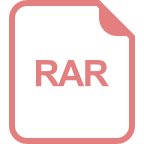
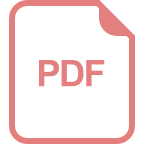




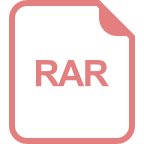
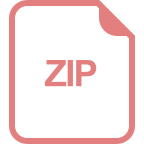
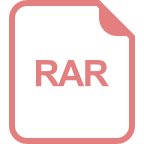
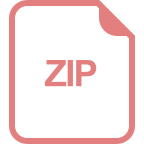



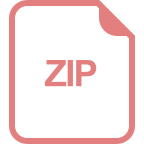
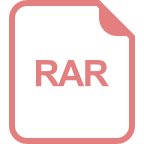
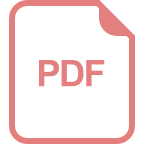
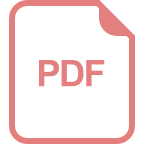
