pyqt QTableWidget 标题栏设置checkbox选择框
时间: 2024-10-09 22:05:34 浏览: 110
PyQt中的QTableWidget允许您自定义表头,包括添加复选框。要实现标题栏设置checkbox选择框,你可以按照以下步骤操作:
1. 创建QTableWidget实例,并设置列数:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTableWidgetItem, QVBoxLayout, QWidget
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QFont
class MyWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('QTableWidget with Checkbox')
self.table = QTableWidget(self)
self.init_table()
def init_table(self):
self.table.setColumnCount(4)
self.table.setHorizontalHeaderLabels(['Checkbox', 'Column 1', 'Column 2', 'Column 3'])
```
2. 在表头的第一列插入QTableWidgetItem作为复选框:
```python
checkbox_header = QTableWidgetItem()
checkbox_header.setFlags(Qt.ItemIsUserCheckable | Qt.ItemIsEnabled) # 设置可以被点击并切换状态
checkbox_header.setCheckState(Qt.Unchecked) # 初始状态为未选中
self.table.setHorizontalHeaderItem(0, checkbox_header)
```
3. 通过连接信号处理程序来响应复选框的选中/取消选中事件:
```python
self.table.cellClicked.connect(self.cell_checkbox_clicked)
def cell_checkbox_clicked(self, row, column):
if column == 0: # 只有第一列是复选框
if self.table.item(row, 0).checkState() == Qt.Checked:
print(f"Row {row} is selected")
else:
print(f"Row {row} is deselected")
```
4. 显示窗口:
```python
app = QApplication([])
window = MyWindow()
window.show()
app.exec_()
```
现在,你已经有了一个带有复选框标题栏的QTableWidget,用户可以在每一行的第一列选择或取消选择。
阅读全文
相关推荐







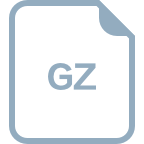










