c++ ,string转LPCWSTR
时间: 2023-10-24 11:04:49 浏览: 258
在C++中,将`std::string`转换为`LPCWSTR`(宽字符指针)可以使用多个方法。以下是两种常见的方法:
方法1: 使用MultiByteToWideChar函数进行转换
```cpp
#include <Windows.h>
#include <string>
LPCWSTR StringToLPCWSTR(const std::string& str) {
int size = MultiByteToWideChar(CP_UTF8, 0, str.c_str(), -1, NULL, 0);
wchar_t* buffer = new wchar_t[size];
MultiByteToWideChar(CP_UTF8, 0, str.c_str(), -1, buffer, size);
return buffer;
}
int main() {
std::string myString = "Hello, World!";
LPCWSTR wideString = StringToLPCWSTR(myString);
// 使用wideString进行操作
delete[] wideString; // 记得释放内存
return 0;
}
```
在上述代码中,我们定义了一个名为`StringToLPCWSTR`的辅助函数,它将`std::string`转换为`LPCWSTR`。该函数使用了`MultiByteToWideChar`函数来进行转换。首先,我们通过调用`MultiByteToWideChar`两次来计算所需的宽字符缓冲区大小,并使用`new`操作符分配相应大小的缓冲区。然后,我们再次调用`MultiByteToWideChar`来执行实际的转换,将字符串拷贝到缓冲区中。最后,我们返回缓冲区的指针,并在使用完后记得使用`delete[]`释放内存。
方法2: 使用std::wstring和c_str方法
```cpp
#include <Windows.h>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::wstring wideString(myString.begin(), myString.end());
LPCWSTR lpwstr = wideString.c_str();
// 使用lpwstr进行操作
return 0;
}
```
在这种方法中,我们首先将`std::string`转换为`std::wstring`,它是一个宽字符的字符串类型。然后,我们可以使用`c_str`方法来获取该宽字符串的指针(`LPCWSTR`类型)。
请注意,在使用这两种方法时,需要确保输入的`std::string`字符串是以UTF-8编码的,因为在Windows中,宽字符以UTF-16编码表示。
希望这些方法能够帮助您将`std::string`转换为`LPCWSTR`。根据您的具体情况,选择适合您需求的方法。
阅读全文
相关推荐
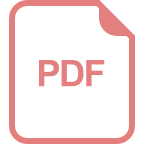
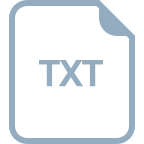









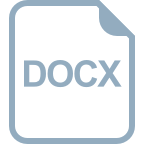





