c++ if else判断一个字母是大写字母、小写字母还是其他字符
时间: 2024-09-24 17:24:43 浏览: 88
在C++中,你可以使用if-else结构以及ASCII码值来判断一个字符是大写字母、小写字母还是其他字符。ASCII码规定了每个字符的整数值,其中大写字母A-Z的编码范围大约是65到90,小写字母a-z的编码范围大约是97到122。
下面是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
int main() {
char ch;
cout << "Enter a character: ";
cin >> ch;
// 判断是否为大写字母
if (ch >= 'A' && ch <= 'Z') {
cout << ch << " is an uppercase letter." << endl;
}
// 判断是否为小写字母
else if (ch >= 'a' && ch <= 'z') {
cout << ch << " is a lowercase letter." << endl;
}
// 其他字符
else {
cout << ch << " is neither uppercase nor lowercase." << endl;
}
return 0;
}
```
在这个程序中,通过比较输入的字符和ASCII码的特定区间,我们可以得出相应的结论。
相关问题
判断一个字符是大写字母、小写字母还是其他字符。c++详细代码
在C++中,你可以使用`ctype`库中的函数来判断一个字符是大写字母、小写字母还是其他字符。下面是一个详细的示例:
```cpp
#include <cctype> // 引入ctype库
int main() {
char ch = 'A'; // 假设我们想检查这个字符
if (isalpha(ch)) { // 检查字符是否是字母
if (isupper(ch)) { // 如果是大写字母
std::cout << "The character is an uppercase letter." << std::endl;
} else if (islower(ch)) { // 否则是小写字母
std::cout << "The character is a lowercase letter." << std::endl;
} else {
std::cout << "The character is neither upper nor lower case, it's another character like digits or special symbols." << std::endl;
}
} else {
std::cout << "The character is not an alphabet." << std::endl;
}
return 0;
}
```
这里,`isalpha()`用于测试字符是否是字母(包括大写和小写),`isupper()`用于测试字符是否为大写字母,`islower()`用于测试字符是否为小写字母。
用c++输入一个字符,判断是不是字母,是大写转换成小写,是小写转换成大写,否则显示不是字母
以下是C知道的回答:
可以使用C语言中的isalpha函数来判断输入的字符是否为字母。如果是大写字母,可以使用tolower函数将其转换为小写字母;如果是小写字母,可以使用toupper函数将其转换为大写字母。如果不是字母,则可以使用printf函数输出"不是字母"的提示信息。以下是示例代码:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char c;
printf("请输入一个字符:");
scanf("%c", &c);
if (isalpha(c)) {
if (isupper(c)) {
c = tolower(c);
} else {
c = toupper(c);
}
printf("转换后的字符为:%c\n", c);
} else {
printf("不是字母\n");
}
return 0;
}
```
希望能帮到您!
阅读全文
相关推荐
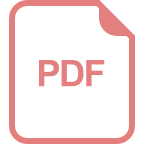
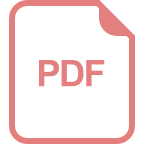
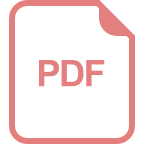













