c语言验证如何验证大小端?
时间: 2024-09-28 17:11:33 浏览: 6
C语言中验证大小端可以通过比较不同类型的内存布局来实现。这里有两种常见的方法:
1. 比较无符号字符数组[^1]:
```c
unsigned char b[] = {0x11}; // 假设这是从网络接收的数据
if (*b == 0x11) {
printf("Little-endian");
} else {
printf("Big-endian");
}
```
这里通过查看第一个字节是否是固定的值(如0x11),推测数据的存储顺序。
2. 使用指针间接访问整数:
```c
int i;
*(char *)&i = 1; // 尝试将1存储到int的第一个字节位置
if (*(char *)&i == 1) {
printf("Little-endiann");
} else {
printf("Big-endiann");
}
```
如果第一个字节确实是1,那么可能是小端模式,因为通常小端机种会先存储最低位。
请注意,这些方法仅适用于特定情况,实际验证时可能需要考虑多种因素,比如结构体内的字段对齐、编译器设置等。在生产环境中,通常使用库函数如`htonl()`、`ntohl()`等来处理网络数据的大小端转换。
相关问题
C语言如何兼容大小端?
C语言本身并不能直接控制大小端兼容性,但是可以通过以下方法实现大小端兼容:
1. 使用无符号整型变量,因为无符号整型变量在任何机器上都是一样的。
2. 使用位运算操作,如左移、右移、与、或等操作,可以直接操作二进制位,不受大小端影响。
3. 使用网络字节序(big-endian)进行数据传输。网络字节序是大端字节序,因此在传输数据时,需要先将数据转换为网络字节序,接收方再将其转换为本地字节序。
4. 使用C语言提供的字节序转换函数,如htons、htonl、ntohs、ntohl等函数,可以将数据在不同大小端之间互相转换。
总之,在C语言中实现大小端兼容需要注意数据类型和数据传输方式等因素,需要根据具体情况进行处理。
C语言结构体内指定大小端
在C语言中,结构体成员的内存布局是由编译器决定的,这可能会受到计算机的大小端影响。在指定结构体成员的大小端时,可以使用特殊的语法来指定成员的对齐方式。
例如,可以使用以下语法来指定结构体成员的大小端:
```c
struct mystruct {
int a;
char b;
int c;
} __attribute__((packed, aligned(1)));
```
在上面的代码中,`__attribute__`是GCC编译器的一个扩展,用于指定结构体成员的对齐方式。`packed`选项指定结构体成员的对齐方式为紧凑对齐,`aligned`选项指定结构体成员的对齐方式为1字节对齐。
使用这种方式可以确保结构体成员的大小端是指定的,但是需要注意的是,这可能会影响结构体成员的性能和内存占用。因此,在实际使用中需要谨慎考虑。
相关推荐
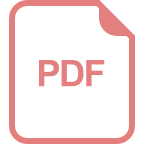
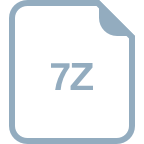
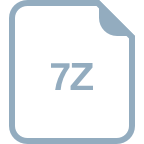












