selenium4中怎么使用firefox_profile.set_preference('browser.helperApps.showOpenOptionForPdfJS', False)
时间: 2024-12-15 21:18:35 浏览: 18
在Selenium v4中,如果你想通过`webdriver.FirefoxProfile()`对象来设置Firefox偏好设置,以便控制PDF.js的行为,例如隐藏显示PDF文件的选项,你可以这样做:
首先,你需要导入`webdriver.FirefoxProfile`模块:
```python
from selenium.webdriver.firefox.options import FirefoxProfile
```
然后创建一个新的`FirefoxProfile`实例:
```python
profile = FirefoxProfile()
```
接下来,你可以使用`set_preference()`方法来设置特定的偏好值。在你的例子中,`browser.helperApps.showOpenOptionForPdfJS`是一个用于控制PDF.js是否显示打开PDF文件选项的偏好。将False传入该方法表示关闭这个选项:
```python
profile.set_preference("browser.helperApps.showOpenOptionForPdfJS", False)
```
最后,在启动Firefox时,你需要把这个自定义的`FirefoxProfile`传递给`webdriver.Firefox()`构造函数:
```python
driver = webdriver.Firefox(profile)
```
这样,每次打开Firefox时,PDF.js的打开选项就会被禁用。
相关问题
firefox selenium插件
### 关于Firefox浏览器兼容的Selenium插件或扩展
对于自动化测试或其他开发目的而言,寻找与Firefox浏览器兼容的Selenium插件或扩展是一项重要工作。当提及到`org.openqa.selenium.firefox.FirefoxDriver.startClient(FirefoxDriver.java:271)`这一部分代码时,这表明正在处理启动Firefox客户端的过程[^1]。
#### 使用GeckoDriver作为Firefox WebDriver
为了使Selenium能够控制Firefox浏览器执行自动化任务,需要依赖名为GeckoDriver的服务端组件。此驱动程序充当着Selenium与Mozilla Firefox之间的桥梁角色,允许通过WebDriver协议发送命令给Firefox实例并接收响应数据。安装GeckoDriver之后,确保其路径已加入系统的环境变量中以便被识别调用。
```bash
# 下载适用于当前平台架构版本的geckodriver二进制文件
wget https://github.com/mozilla/geckodriver/releases/download/v0.33.0/geckodriver-v0.33.0-linux64.tar.gz
# 解压下载包并将可执行文件移动至合适位置
tar -xvzf geckodriver*.tar.gz && sudo mv geckodriver /usr/local/bin/
```
#### 加载自定义配置文件
有时,在运行自动化脚本前可能希望加载特定设置来定制化Firefox行为。可以通过创建一个Profile对象实现这一点;该对象封装了一系列偏好选项以及附加组件列表等信息。下面展示如何利用Python绑定库selenium构建带有预设参数的新profile:
```python
from selenium import webdriver
from selenium.webdriver.firefox.options import Options as FirefoxOptions
from selenium.webdriver.firefox.service import Service as FirefoxService
from selenium.webdriver.firefox.firefox_profile import FirefoxProfile
def setup_firefox_with_custom_settings():
profile = FirefoxProfile()
# 设置一些示例性的首选项
profile.set_preference('browser.download.folderList', 2)
profile.set_preference('browser.helperApps.neverAsk.saveToDisk',
'application/pdf,application/octet-stream')
options = FirefoxOptions()
service = FirefoxService(executable_path='/path/to/geckodriver')
driver = webdriver.Firefox(firefox_profile=profile, options=options,
service=service)
return driver
```
上述方法不仅限于此处列举的具体属性值调整,还可以依据实际需求进一步修改其他方面如启用/禁用JavaScript支持、更改默认主页地址等等[^2]。
selenium 代理
### 如何在 Selenium 中设置和使用代理服务器
#### 创建配置对象并添加代理参数
对于 Chrome 浏览器,在 Python 的 Selenium 库中可以通过 `ChromeOptions` 类来创建配置对象,并向其中添加命令行参数以指定使用的 HTTP 代理服务器地址。
```python
from selenium.webdriver import Chrome, ChromeOptions
options = ChromeOptions()
options.add_argument('--proxy-server=http://代理服务器:端口')
driver = Chrome(options=options)
```
如果需要处理更复杂的场景,比如 HTTPS 或 SOCKS 协议,则可以利用第三方库 `selenium-wire` 来简化这一过程[^2]:
```bash
pip install selenium-wire
```
接着按照如下方式初始化带有自定义代理设置的 WebDriver 实例:
```python
from seleniumwire import webdriver as wirewebdriver
options = {
'proxy': {
'http': 'http://用户名:密码@代理服务器:端口',
'https': 'https://用户名:密码@代理服务器:端口',
'no_proxy': 'localhost,127.0.0.1' # 不走代理的情况下的例外列表
}
}
browser = wirewebdriver.Chrome(seleniumwire_options=options)
```
针对 Firefox 浏览器而言,除了基本相同的步骤外,还需特别关注当涉及到认证型代理时所要做的额外设定。这通常涉及到了一些特定于浏览器内部实现细节方面的调整[^3]:
```python
from selenium.webdriver.firefox.options import Options as FirefoxOptions
firefox_profile = webdriver.FirefoxProfile()
# 设置HTTP/HTTPS代理
firefox_profile.set_preference('network.proxy.type', 1)
firefox_profile.set_preference('network.proxy.http', '代理服务器')
firefox_profile.set_preference('network.proxy.http_port', int(端口号))
firefox_profile.set_preference('network.proxy.ssl', '代理服务器')
firefox_profile.set_preference('network.proxy.ssl_port', int(端口号))
if 需要身份验证:
firefox_profile.set_preference("network.proxy.http.Proxy-Authorization", "Basic "+base64.b64encode(f"{username}:{password}".encode()).decode())
options = FirefoxOptions()
options.profile = firefox_profile
driver = webdriver.Firefox(options=options)
```
以上展示了不同情况下如何为不同的 Web 浏览器配置代理的方法。值得注意的是,具体 API 可能会随着新版本发布而有所变动;因此建议查阅最新版官方文档获得最准确的信息。
阅读全文
相关推荐














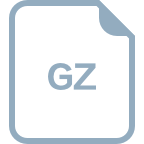

