自定义向量类,满足向量维数可缩放,使用构造函数,析构函数,拷贝构造函数,赋值运算函数,使用异常处理,使用友元函数,使用运算符重载,名字叫MyVector,使用三文件结构
时间: 2024-10-09 22:10:39 浏览: 22
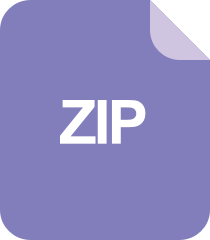
机器学习 支持向量机分类器使用
自定义向量类MyVector,实现动态大小和维度可变,我们可以设计如下的三个文件结构:
1. **myvector.h** (头文件)
```cpp
#ifndef MYVECTOR_H
#define MYVECTOR_H
#include <iostream>
#include <exception>
class MyVector {
private:
int dimensions;
double* data;
public:
MyVector(int initial_capacity);
~MyVector();
MyVector(const MyVector& other); // 拷贝构造函数
MyVector& operator=(const MyVector& other); // 赋值运算符
void resize(int new_dim, double default_value = 0.0);
double& operator[](int index);
double get(int index) const;
void set(int index, double value);
friend std::ostream& operator<<(std::ostream&, const MyVector&);
// 其他友元函数及运算符重载...
private:
void allocate(int capacity);
void deallocate();
};
#endif // MYVECTOR_H
```
2. **myvector.cpp** (实现文件)
```cpp
#include "myvector.h"
MyVector::MyVector(int initial_capacity) : dimensions(1), data(new double[initial_capacity]) {
allocate(initial_capacity);
}
~MyVector() {
deallocate();
}
// ... 实现其他函数
MyVector::MyVector(const MyVector& other) : dimensions(other.dimensions), data(new double[dimensions * other.dimensions]) {
for (int i = 0; i < dimensions * other.dimensions; ++i) {
data[i] = other.data[i];
}
resize(dimensions);
}
// ... 实现赋值运算符和其他函数
void MyVector::allocate(int capacity) {
if (capacity == 0) throw std::invalid_argument("Capacity cannot be zero");
delete[] data;
data = new double[capacity];
}
void MyVector::deallocate() {
delete[] data;
}
// ... 运算符重载和友元函数实现...
```
3. **main.cpp** (示例使用文件)
```cpp
#include "myvector.h"
#include <iostream>
int main() {
try {
MyVector v(3);
v.resize(5); // 可缩放维数
v[0] = 42.0; // 使用索引访问元素
MyVector w(v); // 拷贝构造
w[2] = 99.0;
std::cout << v << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
在这个设计中,我们包含了构造函数、析构函数、拷贝构造函数、赋值运算符、resize方法等,同时通过异常处理机制处理可能出现的问题,并利用友元函数和运算符重载来增强类的功能。
阅读全文
相关推荐
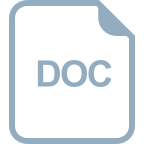
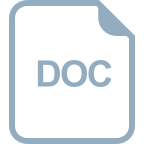

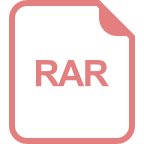
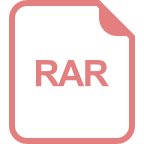
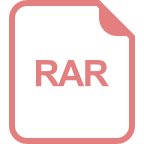
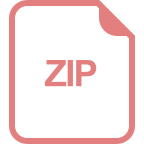
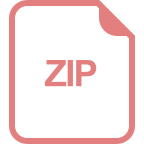
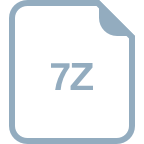
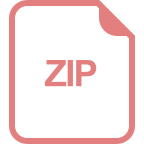
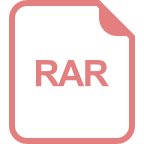
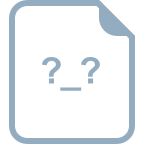
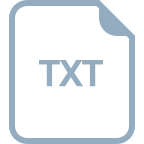
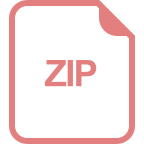
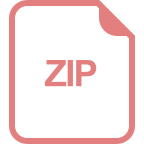
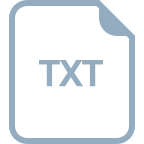
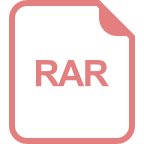
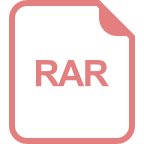