opencv识别正方形
时间: 2023-08-31 22:08:33 浏览: 252
要使用OpenCV来识别正方形,你可以按照以下步骤进行操作:
1. 导入必要的库和模块:
```python
import cv2
import numpy as np
```
2. 读取图像并进行预处理:
```python
image = cv2.imread('your_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
3. 对图像进行边缘检测:
```python
edges = cv2.Canny(gray,50, 150)
```
4. 在边缘图像中查找轮廓:
```python
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
5. 遍历所有轮廓,并筛选出符合条件的正方形轮廓:
```python
squares = []
for contour in contours:
peri = cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, 0.02 * peri, True)
if len(approx) == 4:
x, y, w, h = cv2.boundingRect(approx)
aspectRatio = float(w) / h
if aspectRatio >= 0.9 and aspectRatio <= 1.1:
squares.append(approx)
```
6. 在原始图像上绘制检测到的正方形轮廓:
```python
cv2.drawContours(image, squares, -1, (0, 255, 0), 3)
```
7. 显示结果:
```python
cv2.imshow('Square Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这些步骤将帮助你使用OpenCV来识别并绘制出图像中的正方形轮廓。请注意,这只是一种简单的方法,对于复杂场景中的正方形识别可能需要更复杂的算法和技术。
阅读全文
相关推荐








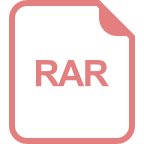
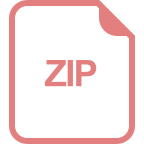
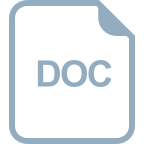
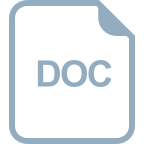
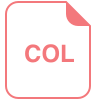
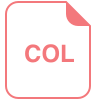
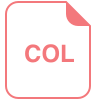
