三个矩阵相乘。第一行输入3个整数a,b,c(都小于100),表示第一个矩阵是a行b列,第二个矩阵是b行c列,第三个矩阵是c行a列,接着下来分别是三个矩阵的数据。输出这三个矩阵相乘的结果,每行a个数据。
时间: 2024-12-06 16:24:49 浏览: 17
由于描述的是一个动态过程,我会为你展示一个基础的伪代码结构,你可以根据这个结构编写C++程序。首先,你需要定义一个函数来处理矩阵乘法,然后读取每个矩阵的值并计算最终结果。
```cpp
#include <vector>
using namespace std;
// 矩阵类,简化版本,只包含必要的部分
class Matrix {
public:
int rows, cols;
vector<vector<int>> data;
Matrix(int a, int b) {
rows = a;
cols = b;
data.resize(rows, vector<int>(cols));
}
void multiply(Matrix &other) {
if (cols != other.rows) {
cerr << "Matrix multiplication not possible." << endl;
return;
}
data.resize(other.cols);
for (int i = 0; i < data.size(); i++) {
for (int j = 0; j < cols; j++) {
data[i][j] = 0;
for (int k = 0; k < other.cols; k++) {
data[i][j] += this->data[i][k] * other.data[k][j];
}
}
}
}
void display() {
for (const auto &row : data) {
for (int num : row) {
cout << num << " ";
}
cout << endl;
}
}
};
int main() {
int a, b, c;
cin >> a >> b >> c; // 获取矩阵维度
Matrix mat1(a, b), mat2(b, c), mat3(c, a);
// 读取并存储矩阵数据
for (int i = 0; i < a && cin >> mat1.data[i][0]; i++) {
for (int j = 1; j < b; j++) {
cin >> mat1.data[i][j];
}
}
for (int i = 0; i < b && cin >> mat2.data[i][0]; i++) {
for (int j = 1; j < c; j++) {
cin >> mat2.data[i][j];
}
}
// 计算矩阵乘法
mat3.multiply(mat2);
mat3.multiply(mat1);
// 输出结果
mat3.display();
return 0;
}
```
注意,这个例子仅适用于连续输入三个矩阵的数据,如果你需要从文件或其他输入源读取,你需要相应地调整输入部分。同时,此代码仅支持标准的矩阵乘法规则,即A(行m列n)乘以B(列n行p)等于C(行m列p)。
阅读全文
相关推荐
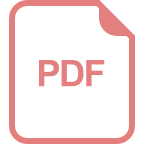
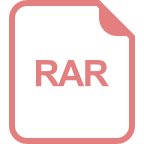
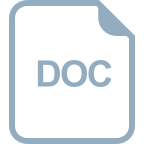















