写一个程序要求输入一个数字将其转化为八进制或者十六进制进行输出,并且以菜单形式,选1或者2来选择转化为八进制或者十六进制。用C语言for循环表示
时间: 2024-09-25 20:04:27 浏览: 39
在C语言中,你可以创建一个简单的命令行程序,通过for循环来接收用户输入并转换成八进制或十六进制。下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
// 转换函数
void to_octal(int num) {
char octal[10];
int i = 0;
while (num > 0) {
octal[i++] = num % 8 + '0';
num /= 8;
}
// 反转字符串以便正确的显示
for (int j = 0; j < i / 2; ++j) {
char temp = octal[j];
octal[j] = octal[i - 1 - j];
octal[i - 1 - j] = temp;
}
printf("Octal representation: %s\n", octal);
}
void to_hexadecimal(int num) {
char hex[10];
int i = 0;
while (num != 0) {
hex[i++] = num % 16 + '0';
if (hex[i - 1] > '9') {
hex[i++] = 'A' + (hex[i - 1] - 'A' - 10);
}
num /= 16;
}
printf("Hexadecimal representation: %s\n", hex);
}
int main() {
int choice, number;
printf("Enter a number: ");
scanf("%d", &number);
while (1) {
printf("\nChoose conversion type:\n1. Octal\n2. Hexadecimal\n");
printf("Enter your choice (1 or 2): ");
scanf("%d", &choice);
switch (choice) {
case 1:
to_octal(number);
break;
case 2:
to_hexadecimal(number);
break;
default:
printf("Invalid choice! Please try again.\n");
break;
}
printf("Do you want to convert another number? (y/n)");
char response;
scanf(" %c", &response);
if (response == 'n' || response == 'N')
break;
}
return 0;
}
```
在这个程序中,我们首先定义了两个函数`to_octal`和`to_hexadecimal`分别用于将十进制数转换为八进制和十六进制。然后在主循环中,根据用户的输入选择相应的转换并询问是否继续。
阅读全文
相关推荐
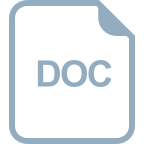
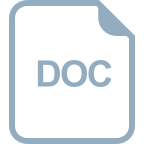
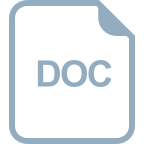


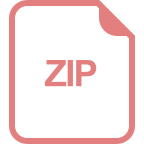
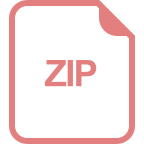
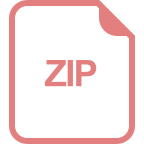
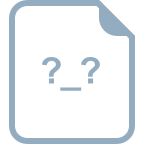
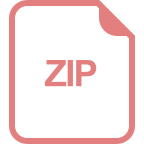
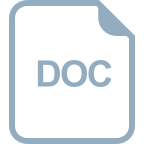
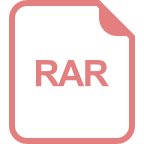
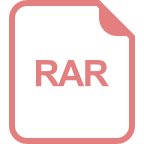
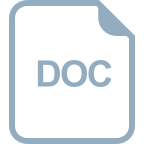
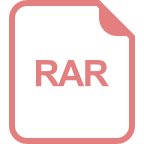
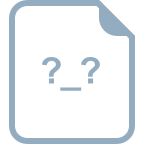
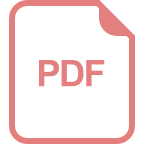
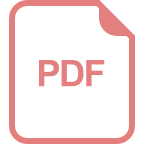
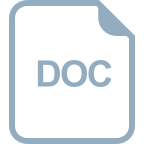