c++凯撒密码加密解密代码运行图
时间: 2024-10-09 11:12:44 浏览: 41
在C++中实现凯撒密码的加密和解密,你可以创建两个函数,一个用于加密,另一个用于解密,它们的核心都是对输入字符串的每个字符进行位移。这里是一个简单的例子:
```cpp
#include <iostream>
#include <string>
// 函数定义,偏移量设为3作为示例
std::string caesar_cipher_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 只处理英文字母
char shifted = 'A' <= c && c <= 'Z' ? static_cast<char>((c - 'A' + shift) % 26 + 'A') :
static_cast<char>((c - 'a' + shift) % 26 + 'a');
ciphertext += shifted;
} else {
ciphertext += c; // 非字母字符不变
}
}
return ciphertext;
}
std::string caesar_cipher_decrypt(const std::string& ciphertext, int shift) {
return caesar_cipher_encrypt(ciphertext, -shift);
}
int main() {
std::string input = "Hello, World!";
int shift = 3;
std::string encrypted = caesar_cipher_encrypt(input, shift);
std::cout << "Encrypted text: " << encrypted << std::endl;
std::string decrypted = caesar_cipher_decrypt(encrypted, shift);
std::cout << "Decrypted text: " << decrypted << std::endl;
return 0;
}
```
这个程序首先定义了一个加密函数`caesar_cipher_encrypt`,接收一个字符串和偏移量,然后遍历字符串中的每个字符,如果是字母则按偏移量进行循环移位,最后返回加密后的字符串。解密函数`caesar_cipher_decrypt`则是对加密后的字符串做相反的操作。
运行这个程序,你会看到输入字符串经过凯撒密码加密后以及解密后的效果。
阅读全文
相关推荐
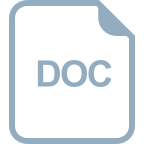
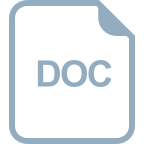
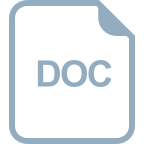
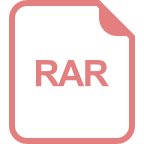
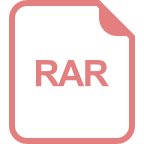
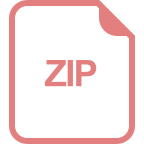
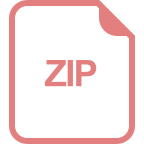
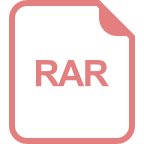
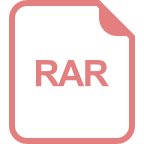
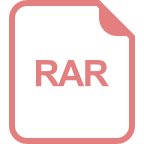
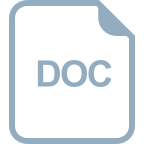
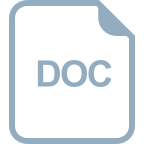
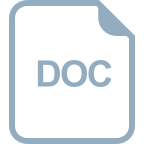
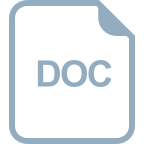
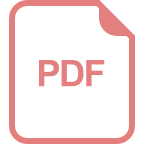
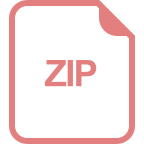
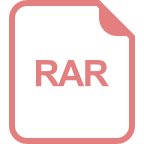
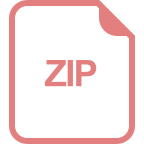
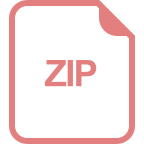