用C++写一个简单的QT上位机程序
时间: 2023-10-20 17:05:36 浏览: 113
下面是一个用C++和QT编写的简单上位机程序的示例:
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QPushButton>
#include <QLabel>
#include <QVBoxLayout>
#include <QDebug>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr)
: QMainWindow(parent)
{
// 创建一个按钮
QPushButton *button = new QPushButton("Click me", this);
connect(button, &QPushButton::clicked, this, &MainWindow::onButtonClicked);
// 创建一个标签
label = new QLabel("Button not clicked yet", this);
// 创建一个垂直布局,并将按钮和标签添加进去
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(button);
layout->addWidget(label);
// 创建一个主窗口,并将布局设置为主窗口的中心部件
QWidget *centralWidget = new QWidget(this);
centralWidget->setLayout(layout);
setCentralWidget(centralWidget);
}
private slots:
void onButtonClicked()
{
qDebug() << "Button clicked";
label->setText("Button clicked");
}
private:
QLabel *label;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow window;
window.show();
return app.exec();
}
#include "main.moc"
```
上述代码使用了QT的基本类,创建了一个简单的上位机程序窗口。窗口中包含一个按钮和一个标签,当按钮被点击时,标签的文本会更新为"Button clicked"。
在main函数中,创建了QT应用程序对象并实例化了MainWindow类的对象,然后执行应用程序的主事件循环。
请注意,上述代码应保存为一个.cpp文件,并使用QT的qmake等工具进行编译和构建。在编写和构建QT程序时,需要配置和链接QT库,并确保正确设置了QT环境。
这只是一个简单的示例,您可以根据自己的需求进行扩展和修改,添加更多的界面元素和功能。请参考QT的官方文档和示例代码,以获取更详细的指导和参考。
阅读全文
相关推荐

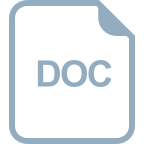
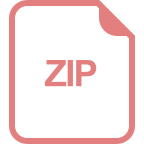
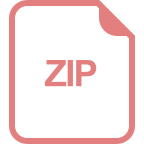
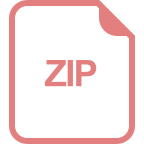
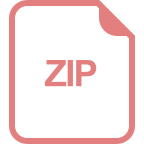
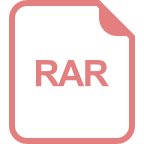
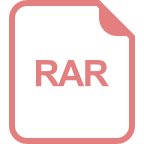
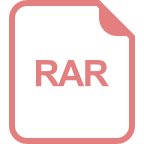
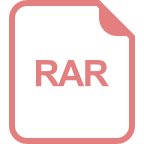
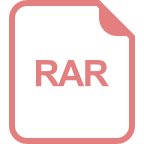
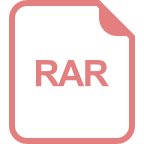
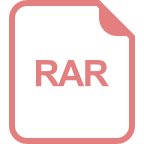
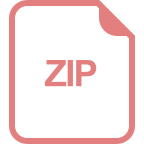
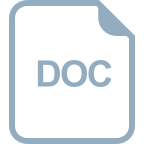
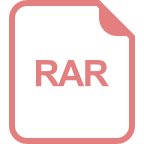