java多线程复杂样例
时间: 2024-05-24 12:09:20 浏览: 12
Java多线程的复杂样例有很多,以下是一个简单的生产者-消费者模型的实现,其中包括了线程同步、等待通知、多线程并发等问题:
```java
public class ProducerConsumerExample {
private static final int BUFFER_SIZE = 10;
private final List<Integer> buffer = new ArrayList<>(BUFFER_SIZE);
private final Random random = new Random();
public void produce() throws InterruptedException {
while (true) {
synchronized (this) {
while (buffer.size() == BUFFER_SIZE) {
wait();
}
int number = random.nextInt();
buffer.add(number);
System.out.println("Produced: " + number);
notifyAll();
}
}
}
public void consume() throws InterruptedException {
while (true) {
synchronized (this) {
while (buffer.size() == 0) {
wait();
}
int number = buffer.remove(0);
System.out.println("Consumed: " + number);
notifyAll();
}
}
}
public static void main(String[] args) {
ProducerConsumerExample example = new ProducerConsumerExample();
Thread producerThread = new Thread(() -> {
try {
example.produce();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
Thread consumerThread = new Thread(() -> {
try {
example.consume();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
producerThread.start();
consumerThread.start();
}
}
```
上述代码中,生产者和消费者共享一个缓冲区`buffer`,当缓冲区为空时,消费者线程进入等待状态,当缓冲区已满时,生产者线程进入等待状态。当一个线程完成操作后,通过调用`notifyAll()`唤醒其他等待线程。这样就能够保证生产者和消费者交替执行,并且不会出现资源竞争和死锁等问题。
相关推荐
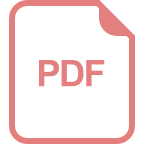
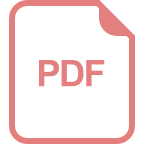














