golang烟花代码
时间: 2024-04-10 20:26:13 浏览: 183
Golang烟花代码是一种使用Go语言编写的模拟烟花爆炸效果的程序。它通过使用Golang的图形库和并发机制,实现了烟花在屏幕上绽放的效果。
以下是一个简单的Golang烟花代码示例:
```go
package main
import (
"fmt"
"math/rand"
"time"
"github.com/fogleman/gg"
)
const (
width = 800
height = 600
)
type Particle struct {
X, Y float64
VX, VY float64
Life int
Color string
Sparkles []Sparkle
}
type Sparkle struct {
X, Y float64
VX, VY float64
Life int
Color string
}
func main() {
dc := gg.NewContext(width, height)
dc.SetRGB(0, 0, 0)
dc.Clear()
particles := make([]Particle, 0)
for i := 0; i < 100; i++ {
p := Particle{
X: width / 2,
Y: height,
VX: rand.Float64()*6 - 3,
VY: -rand.Float64()*5 - 5,
Life: rand.Intn(60) + 60,
Color: fmt.Sprintf("#%06x", rand.Intn(0xffffff)),
}
particles = append(particles, p)
}
for len(particles) > 0 {
dc.Clear()
for i := len(particles) - 1; i >= 0; i-- {
p := &particles[i]
dc.SetRGB255(parseColor(p.Color))
dc.DrawPoint(p.X, p.Y, 1)
p.X += p.VX
p.Y += p.VY
p.VY += 0.1
p.Life--
if p.Life <= 0 {
particles = append(particles[:i], particles[i+1:]...)
continue
}
for j := len(p.Sparkles) - 1; j >= 0; j-- {
s := &p.Sparkles[j]
dc.SetRGB255(parseColor(s.Color))
dc.DrawPoint(s.X, s.Y, 1)
s.X += s.VX
s.Y += s.VY
s.VY += 0.1
s.Life--
if s.Life <= 0 {
p.Sparkles = append(p.Sparkles[:j], p.Sparkles[j+1:]...)
}
}
if rand.Float64() < 0.02 {
s := Sparkle{
X: p.X,
Y: p.Y,
VX: rand.Float64()*6 - 3,
VY: rand.Float64()*6 - 3,
Life: rand.Intn(20) + 20,
Color: fmt.Sprintf("#%06x", rand.Intn(0xffffff)),
}
p.Sparkles = append(p.Sparkles, s)
}
}
dc.Stroke()
dc.SavePNG("fireworks.png")
time.Sleep(time.Millisecond * 10)
}
}
func parseColor(color string) (int, int, int) {
var r, g, b int
fmt.Sscanf(color, "#%02x%02x%02x", &r, &g, &b)
return r, g, b
}
```
这段代码使用了第三方库`github.com/fogleman/gg`来进行图形绘制。它模拟了烟花的爆炸效果,通过不断更新粒子的位置和颜色,实现了烟花在屏幕上绽放的效果。
阅读全文
相关推荐
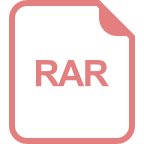
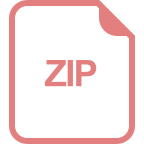
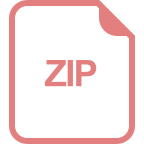
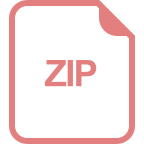
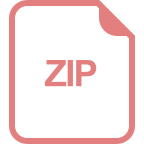
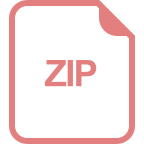
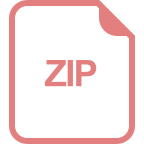
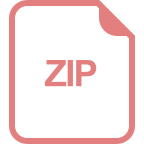
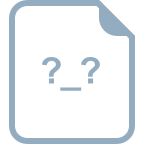
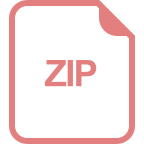
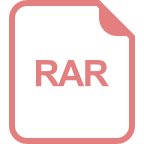

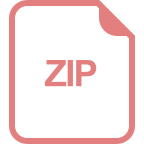
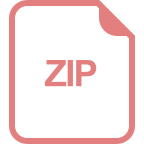
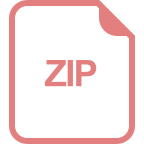