能基于linemod算法,使用c++语言,在clion下,写出一个模板图片与视频的模板匹配代码吗?
时间: 2024-04-14 17:25:36 浏览: 128
当然可以!以下是一个基于LineMod算法的模板匹配代码示例,使用C++语言编写,并在CLion下运行:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
#include <opencv2/linemod.hpp>
using namespace std;
using namespace cv;
void drawResponse(const std::vector<cv::linemod::Match>& matches, cv::Mat& dst, const std::vector<cv::Ptr<cv::linemod::Template>>& templates, const cv::Ptr<cv::linemod::Detector>& detector);
int main()
{
// 读取模板图片
cv::Mat templateImage = cv::imread("template.png", 0);
if (templateImage.empty())
{
std::cout << "Failed to read template image!" << std::endl;
return -1;
}
// 创建模板对象
std::vector<cv::Mat> templateImages;
templateImages.push_back(templateImage);
cv::Ptr<cv::linemod::Detector> detector = cv::linemod::getDefaultLINE();
detector->addTemplate(templateImages, "template");
// 读取视频
cv::VideoCapture cap("video.mp4");
if (!cap.isOpened())
{
std::cout << "Failed to open video!" << std::endl;
return -1;
}
// 创建窗口
cv::namedWindow("Template Matching", cv::WINDOW_NORMAL);
// 进行模板匹配
cv::Mat frame;
while (cap.read(frame))
{
// 转换为灰度图像
cv::Mat gray;
cv::cvtColor(frame, gray, cv::COLOR_BGR2GRAY);
// 运行模板匹配
std::vector<cv::linemod::Match> matches;
detector->match(gray, 80, matches);
// 绘制匹配结果
drawResponse(matches, frame, detector->getTemplates("template"), detector);
// 显示匹配结果
cv::imshow("Template Matching", frame);
// 按下ESC键退出
if (cv::waitKey(1) == 27)
break;
}
// 释放资源
cv::destroyAllWindows();
cap.release();
return 0;
}
void drawResponse(const std::vector<cv::linemod::Match>& matches, cv::Mat& dst, const std::vector<cv::Ptr<cv::linemod::Template>>& templates, const cv::Ptr<cv::linemod::Detector>& detector)
{
for (size_t i = 0; i < matches.size(); ++i)
{
const cv::linemod::Match& match = matches[i];
const std::vector<cv::linemod::Template>& classTemplates = templates[match.class_id]->templates;
for (size_t j = 0; j < classTemplates.size(); ++j)
{
cv::Mat display = dst.clone();
cv::Rect rect = classTemplates[j].rect;
cv::rectangle(display, rect, cv::Scalar(0, 255, 255));
cv::Point center(match.x, match.y);
cv::circle(display, center, 5, cv::Scalar(0, 0, 255), 2);
cv::imshow("Template Matching", display);
}
}
}
```
请确保安装了OpenCV库,并将示例代码中的`template.png`和`video.mp4`替换为你自己的模板图片和视频文件路径。你可以使用CLion来编译和运行这段代码。
注意:这只是一个简单的示例,实际使用中可能需要进行更多的参数调整和优化。希望对你有帮助!
阅读全文
相关推荐
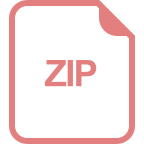
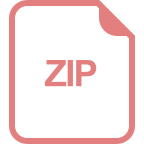
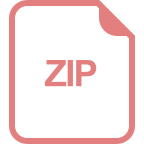












