yaml to xml
时间: 2023-11-25 21:06:39 浏览: 62
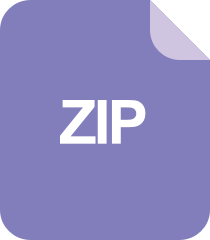
yaml-json-xml-converter-action:可交互转换YAMLJSONXML文件格式的GitHub操作
To convert YAML to XML, you can use a programming language that supports both YAML and XML parsing. Here's an example in Python using the PyYAML and xml.etree.ElementTree libraries:
```python
import yaml
import xml.etree.ElementTree as ET
def yaml_to_xml(yaml_data):
# Load YAML data
data = yaml.safe_load(yaml_data)
# Create the root XML element
root = ET.Element("root")
# Recursively convert YAML to XML
def convert(data, parent):
if isinstance(data, dict):
for key, value in data.items():
element = ET.SubElement(parent, key)
convert(value, element)
elif isinstance(data, list):
for item in data:
convert(item, parent)
else:
parent.text = str(data)
# Convert YAML to XML
convert(data, root)
# Create the XML tree and return it as a string
tree = ET.ElementTree(root)
return ET.tostring(root, encoding="unicode")
# Example usage
yaml_data = """
person:
name: John Doe
age: 30
"""
xml_data = yaml_to_xml(yaml_data)
print(xml_data)
```
This code defines a `yaml_to_xml` function that takes a YAML string as input and returns the corresponding XML string. It uses the PyYAML library to parse the YAML data and xml.etree.ElementTree library to create the XML structure. The function recursively converts the YAML data into XML elements and attributes.
阅读全文
相关推荐
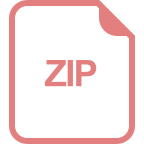
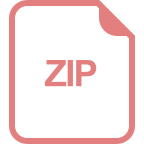
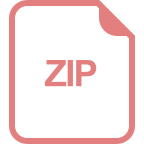

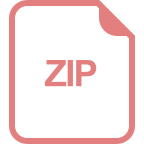
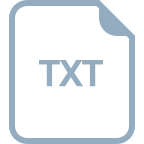
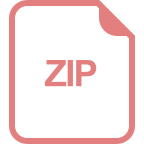
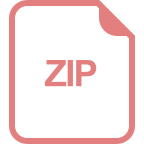
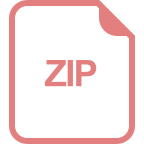
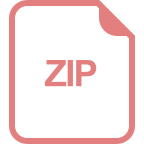
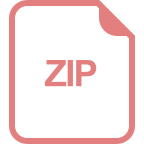





