使用结构体储存学生信息(包括学号,姓名,,3门课程成绩,总分),要求实现对学生信息修改和删除操作,最多50名学生。
时间: 2024-09-08 19:01:39 浏览: 96
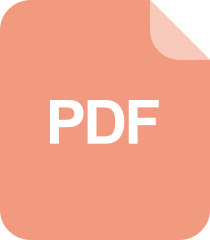
C语言利用结构体数组实现学生成绩管理系统

在C语言中,我们可以使用结构体(struct)来存储学生的个人信息和成绩数据。首先,我们需要定义一个名为`Student`的结构体,包含学号、姓名以及三个成绩变量。然后创建一个数组或者链表来存放最多50名学生的信息。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct {
int id; // 学号
char name[50]; // 姓名
int score1; // 第一门课成绩
int score2; // 第二门课成绩
int score3; // 第三门课成绩
int total_score; // 总分
} Student;
// 功能:添加学生
void add_student(Student students[], int* count, const char* name, int scores[]) {
Student new_student;
new_student.id = (*count) + 1;
strcpy(new_student.name, name);
new_student.score1 = scores[0];
new_student.score2 = scores[1];
new_student.score3 = scores[2];
new_student.total_score = new_student.score1 + new_student.score2 + new_student.score3;
students[*count] = new_student;
(*count)++;
}
// 功能:修改学生信息
void update_student(Student* student, int id, int new_score1, int new_score2, int new_score3) {
if (student[id - 1].id == id) {
student[id - 1].score1 = new_score1;
student[id - 1].score2 = new_score2;
student[id - 1].score3 = new_score3;
student[id - 1].total_score = student[id - 1].score1 + student[id - 1].score2 + student[id - 1].score3;
}
}
// 功能:删除学生
void delete_student(Student* students, int count, int id) {
if (id > 0 && id <= count) {
memmove(&students[id], &students[id + 1], sizeof(Student) * (count - id));
count--;
}
}
// 主函数示例
int main() {
int max_students = 50;
Student students[max_students];
int count = 0;
// 添加学生信息...
// 更新和删除学生...
return 0;
}
```
阅读全文
相关推荐
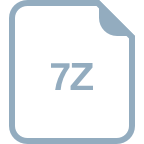
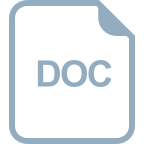










