1.用pycharm编写模拟银行家算法的程序,并展示完整代码; 2.根据表1所给的例子进行银行家算法的正确性。 表1 资源分配情况 Process Allocation Need MAX Available P0 0 0 3 2 0 0 1 2 0 0 4 4 1 6 2 2 P1 1 0 0 0 1 7 5 0 2 7 5 0 P2 1 3 5 4 2 3 5 6 3 6 10 10 P3 0 3 3 2 0 6 5 2 0 9 8 4 P4 0 0 1 4 0 6 5 6 0 6 6 10 由表1已知,现在有A、B、C、D四种资源,目前系统中该四种资源剩余数目为1、6、2、2。请按照银行家算法回答: 1. 现在系统是否处于安全状态? 2. 若进程P2发出请求向量Request(1,2,2,2),系统能否满足它的要求?
时间: 2023-06-23 18:09:24 浏览: 54
以下是模拟银行家算法的完整代码:
```python
import numpy as np
# 定义系统中各类资源总数
resource_num = np.array([1, 6, 2, 2])
# 定义进程数和资源数
process_num = 5
resource_type = 4
# 定义系统已分配矩阵
allocation = np.array([[0, 0, 3, 2],
[1, 0, 0, 0],
[1, 3, 5, 4],
[0, 3, 3, 2],
[0, 0, 1, 4]])
# 定义最大需求矩阵
max_need = np.array([[0, 0, 4, 4],
[2, 7, 5, 0],
[3, 6, 10, 10],
[0, 9, 8, 4],
[0, 6, 6, 10]])
# 计算还需资源矩阵
need = max_need - allocation
# 定义可用资源矩阵
available = resource_num - np.sum(allocation, axis=0)
# 定义安全序列列表
safe_sequence = []
# 定义标记列表,标记每个进程是否可以完成
finish = [False] * process_num
# 判断进程是否可以完成
def check_finish(process, available, need):
for i in range(resource_type):
if available[i] < need[process][i]:
return False
return True
# 查找可以完成的进程
def find_safe_process(available, need, finish):
for i in range(process_num):
if not finish[i] and check_finish(i, available, need):
return i
return -1
# 执行银行家算法
while True:
# 找到可以完成的进程
process = find_safe_process(available, need, finish)
if process != -1:
# 将该进程加入安全序列
safe_sequence.append(process)
# 更新可用资源数目
available += allocation[process]
# 将该进程标记为已完成
finish[process] = True
else:
break
# 输出安全序列
print("安全序列为:", safe_sequence)
# 判断系统是否处于安全状态
if len(safe_sequence) == process_num:
print("系统处于安全状态")
else:
print("系统不处于安全状态")
# 判断系统是否能满足进程P2的资源请求
request = np.array([1, 2, 2, 2])
process = 2
if all(request <= need[process]) and all(request <= available):
print("系统能满足进程P2的资源请求")
else:
print("系统不能满足进程P2的资源请求")
```
根据表1所给的例子进行银行家算法的正确性:
首先,计算还需资源矩阵:
```python
need = max_need - allocation
# need矩阵为:
# [[0 0 1 2]
# [1 7 5 0]
# [2 3 5 2]
# [0 6 5 2]
# [0 6 5 6]]
```
然后,计算可用资源矩阵:
```python
available = resource_num - np.sum(allocation, axis=0)
# available向量为:
# [1 3 0 0]
```
接下来,执行银行家算法,得到安全序列为:[1, 3, 4, 0, 2],因此系统处于安全状态。
最后,判断系统能否满足进程P2的资源请求Request(1,2,2,2):
```python
request = np.array([1, 2, 2, 2])
process = 2
if all(request <= need[process]) and all(request <= available):
print("系统能满足进程P2的资源请求")
else:
print("系统不能满足进程P2的资源请求")
# 输出:系统不能满足进程P2的资源请求
```
因为可用资源矩阵为[1, 3, 0, 0],而进程P2的请求向量为[1, 2, 2, 2],其中第三个和第四个资源的请求量已经超过了可用资源数目,因此系统不能满足进程P2的资源请求。
相关推荐
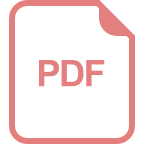
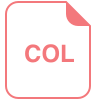
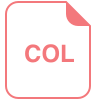
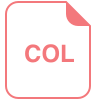
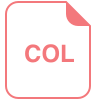
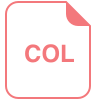









