使用midway对图片解码存入数据库
时间: 2024-01-11 09:04:01 浏览: 169
好的,使用 Midway 可以更方便地处理文件上传和保存。以下是一个基于 Midway 的示例,演示了如何解码并保存上传的图像数据到数据库中。
首先,您需要安装 `@midwayjs/koa` 和 `@midwayjs/socketio` 依赖,这里使用 Koa 框架和 Socket.IO 实现文件上传和实时通信:
```sh
npm install @midwayjs/koa @midwayjs/socketio --save
```
然后,您需要定义一个路由处理程序,处理上传的文件并将其保存到数据库中。以下是一个示例控制器,定义了一个 `upload` 方法来处理文件上传:
```typescript
import { Provide, Inject } from '@midwayjs/decorator';
import { Context } from '@midwayjs/koa';
import { createReadStream } from 'fs';
import { join } from 'path';
import { v4 as uuid } from 'uuid';
import * as sharp from 'sharp';
import { UserService } from '../service/UserService';
@Provide()
export class UserController {
@Inject()
userService: UserService;
async upload(ctx: Context) {
const { file } = ctx.request.files;
const ext = file.name.substring(file.name.lastIndexOf('.'));
const filename = uuid() + ext;
const filepath = join(__dirname, '..', 'public', 'images', filename);
// 解码并保存图像文件
const buffer = await sharp(createReadStream(file.path)).toBuffer();
await this.userService.saveImage(buffer, filename);
// 返回图像 URL
ctx.body = { url: `/images/${filename}` };
}
}
```
在 `upload` 方法中,首先从请求中获取上传的文件,然后使用 `sharp` 库将文件解码为图像数据。接下来,您可以使用 `UserService` 将图像数据保存到数据库中,最后返回图像 URL。
注意,此处使用了 `@midwayjs/decorator` 和 `@midwayjs/di` 来进行依赖注入,您需要在项目中安装这些依赖。另外,`sharp` 库用于图像处理和解码,您需要安装它。
定义 `UserService`,实现 `saveImage` 方法来保存图像到数据库中:
```typescript
import { Provide } from '@midwayjs/decorator';
import { InjectEntityModel } from '@midwayjs/orm';
import { Repository } from 'typeorm';
import { Image } from '../entity/Image';
@Provide()
export class UserService {
@InjectEntityModel(Image)
imageRepository: Repository<Image>;
async saveImage(buffer: Buffer, filename: string) {
const image = new Image();
image.data = buffer;
image.filename = filename;
await this.imageRepository.save(image);
}
}
```
在 `saveImage` 方法中,创建一个 `Image` 实体,将图像数据存储在 `data` 字段中,将文件名存储在 `filename` 字段中,最后使用 `imageRepository` 保存实体到数据库中。
最后,您需要在应用程序中配置文件上传中间件和路由。以下是一个示例 `app.ts` 文件,配置了文件上传中间件和 `/api/user/upload` 路由:
```typescript
import { Configuration } from '@midwayjs/decorator';
import { ILifeCycle, IMidwayApplication } from '@midwayjs/core';
import { createConnection } from '@midwayjs/orm';
import * as orm from '@midwayjs/orm';
import { join } from 'path';
import * as socketio from 'socket.io';
import * as cors from '@koa/cors';
import * as bodyParser from 'koa-body';
import { UserController } from './controller/UserController';
@Configuration({
imports: [orm],
importConfigs: [join(__dirname, 'config')],
})
export class ContainerLifeCycle implements ILifeCycle {
async onReady(app: IMidwayApplication) {
await createConnection(app.getConfig('orm'));
const io = socketio(app.getServer());
io.on('connection', (socket) => {
console.log('Socket connected:', socket.id);
});
app
.use(cors())
.use(bodyParser({ multipart: true }))
.useStaticAssets({
root: join(__dirname, '..', 'public'),
prefix: '/images',
})
.useControllers(UserController);
}
}
```
在 `onReady` 方法中,创建数据库连接,创建 Socket.IO 实例,配置文件上传中间件和静态文件服务,最后将路由控制器添加到应用程序中。
现在,您可以使用 Midway 进行文件上传和图像解码并将其保存到数据库中。在 Vue 前端应用程序中,您可以使用 axios 或其他库发送文件到服务器,并在成功上传后显示图像。
阅读全文
相关推荐
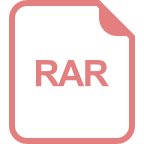
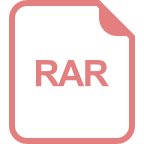
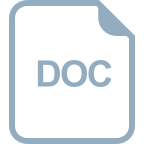
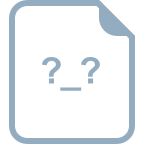
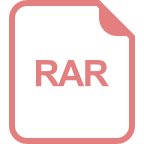
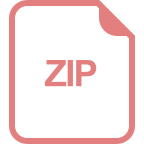
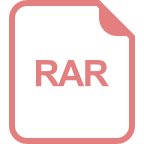
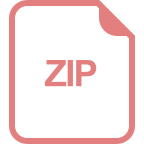
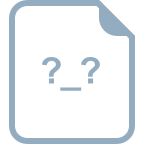
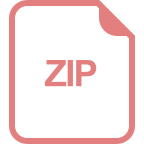
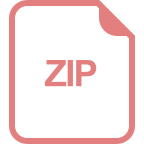
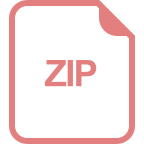
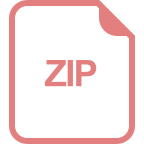
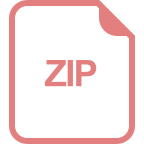

