使用JAVA事件处理实现双人简易聊天窗口,并添加重置按钮
时间: 2023-07-16 14:12:42 浏览: 218
下面是一个使用JAVA事件处理实现双人简易聊天窗口,并添加重置按钮的示例代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ChatWindow extends JFrame implements ActionListener {
private JTextArea chatArea;
private JTextField inputField;
private JButton sendButton;
private JButton resetButton;
public ChatWindow() {
super("Chat Window");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
panel.setLayout(new BorderLayout());
chatArea = new JTextArea();
chatArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(chatArea);
panel.add(scrollPane, BorderLayout.CENTER);
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new BorderLayout());
inputField = new JTextField();
inputField.addActionListener(this);
inputPanel.add(inputField, BorderLayout.CENTER);
sendButton = new JButton("Send");
sendButton.addActionListener(this);
inputPanel.add(sendButton, BorderLayout.EAST);
resetButton = new JButton("Reset");
resetButton.addActionListener(this);
inputPanel.add(resetButton, BorderLayout.WEST);
panel.add(inputPanel, BorderLayout.SOUTH);
setContentPane(panel);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == sendButton || e.getSource() == inputField) {
String text = inputField.getText().trim();
if (!text.isEmpty()) {
chatArea.append("> " + text + "\n");
inputField.setText("");
}
} else if (e.getSource() == resetButton) {
chatArea.setText("");
inputField.setText("");
}
}
public static void main(String[] args) {
ChatWindow chatWindow = new ChatWindow();
chatWindow.setVisible(true);
}
}
```
这个示例代码创建了一个窗口,包括一个文本区域用于显示聊天记录,一个文本框用于输入聊天内容,一个发送按钮用于发送消息,一个重置按钮用于清空聊天记录和输入框。当用户点击发送按钮或者按下回车键时,程序会检查输入框中的内容是否为空,如果不为空,则将内容显示到聊天记录中,并清空输入框;当用户点击重置按钮时,程序会清空聊天记录和输入框。
阅读全文
相关推荐
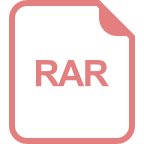
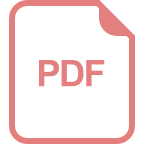
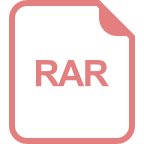
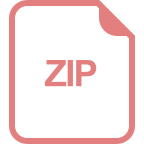
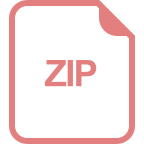
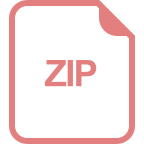
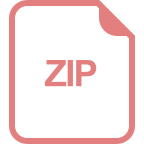
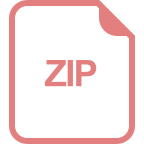
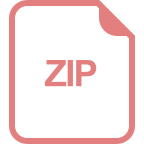
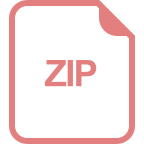