贪心算法的板材排版C#代码
时间: 2023-10-19 12:04:23 浏览: 129
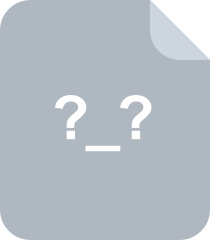
贪心算法 C# 实现
以下是使用贪心算法实现的板材排版的 C# 代码示例:
```csharp
using System;
using System.Collections.Generic;
public class Board
{
public double Width { get; set; }
public double Height { get; set; }
public Board(double width, double height)
{
Width = width;
Height = height;
}
public override string ToString()
{
return $"{Width} x {Height}";
}
}
public class Piece
{
public double Width { get; set; }
public double Height { get; set; }
public Piece(double width, double height)
{
Width = width;
Height = height;
}
public override string ToString()
{
return $"{Width} x {Height}";
}
}
public class BoardCutting
{
public static List<Board> CutBoards(List<Piece> pieces, Board board)
{
List<Board> boards = new List<Board>();
List<Piece> sortedPieces = SortPieces(pieces); // 按面积从大到小排序
while (sortedPieces.Count > 0)
{
Board newBoard = new Board(board.Width, board.Height);
double areaLeft = board.Width * board.Height;
foreach (Piece piece in sortedPieces)
{
if (piece.Width <= newBoard.Width && piece.Height <= newBoard.Height)
{
newBoard.Width -= piece.Width;
areaLeft -= piece.Width * piece.Height;
if (piece.Height > newBoard.Height)
{
newBoard.Height = piece.Height;
}
}
}
if (areaLeft == 0)
{
boards.Add(newBoard);
sortedPieces.RemoveAll(p => p.Width <= newBoard.Width && p.Height <= newBoard.Height);
}
else
{
break;
}
}
return boards;
}
private static List<Piece> SortPieces(List<Piece> pieces)
{
return pieces
.OrderByDescending(p => p.Width * p.Height)
.ToList();
}
}
public class Example
{
public static void Main()
{
Board board = new Board(240, 120);
List<Piece> pieces = new List<Piece>
{
new Piece(100, 80),
new Piece(80, 50),
new Piece(60, 40),
new Piece(40, 30)
};
List<Board> boards = BoardCutting.CutBoards(pieces, board);
Console.WriteLine($"需要的板数量:{boards.Count}");
boards.ForEach(b => Console.WriteLine($"板的尺寸:{b}"));
}
}
```
上述代码中的 `Board` 类表示一块板材,包括宽度和高度两个属性;`Piece` 类表示一块需切割的木板,也包括宽度和高度两个属性。 `BoardCutting` 类包含了实现板材排版的 `CutBoards` 方法,该方法接收一个需切割的木板列表和一个板材对象作为参数,返回使用的板材列表。 `SortPieces` 方法用于对需切割的木板列表按照面积从大到小排序,以便优先使用大的木板进行切割。
在 `Main` 方法中,我们创建了一个板材对象和一个需切割的木板列表,并调用 `CutBoards` 方法进行排版,最后输出使用的板材数量和每个板材的尺寸。
阅读全文
相关推荐
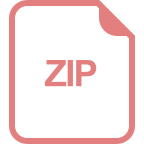
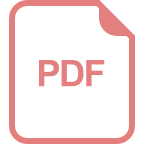
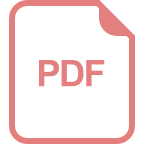
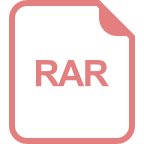
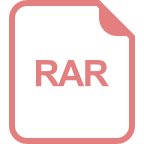
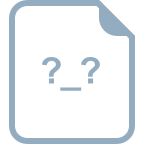
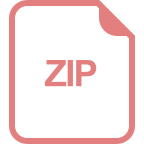
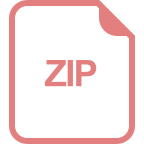
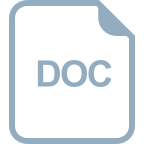
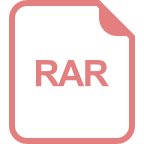
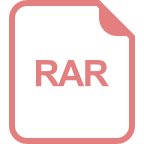
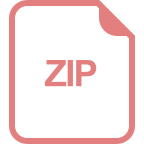
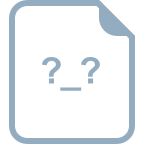