用c使用lzma sdk 22.01压缩文件
时间: 2024-05-10 15:19:26 浏览: 197
以下是使用LZMA SDK 22.01压缩文件的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <lzma.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <input_file> <output_file>\n", argv[0]);
return 1;
}
// 打开输入文件
FILE* f_in = fopen(argv[1], "rb");
if (!f_in) {
printf("Failed to open input file: %s\n", argv[1]);
return 1;
}
// 打开输出文件
FILE* f_out = fopen(argv[2], "wb");
if (!f_out) {
printf("Failed to open output file: %s\n", argv[2]);
fclose(f_in);
return 1;
}
// 初始化压缩器
lzma_stream strm = LZMA_STREAM_INIT;
lzma_options_lzma opt_lzma2;
lzma_lzma_preset(&opt_lzma2, LZMA_PRESET_DEFAULT);
lzma_ret ret = lzma_stream_encoder(&strm, &opt_lzma2, LZMA_CHECK_CRC64);
if (ret != LZMA_OK) {
printf("Failed to initialize encoder: %d\n", ret);
fclose(f_in);
fclose(f_out);
return 1;
}
// 读取并压缩数据
uint8_t in_buf[BUFSIZ];
uint8_t out_buf[BUFSIZ];
size_t in_pos = 0, in_size = 0;
size_t out_pos = 0, out_size = 0;
while ((in_size = fread(in_buf, 1, BUFSIZ, f_in)) > 0) {
strm.next_in = in_buf;
strm.avail_in = in_size;
do {
strm.next_out = out_buf;
strm.avail_out = BUFSIZ;
ret = lzma_code(&strm, LZMA_RUN);
if (ret != LZMA_OK && ret != LZMA_STREAM_END) {
printf("Compression failed: %d\n", ret);
fclose(f_in);
fclose(f_out);
lzma_end(&strm);
return 1;
}
out_size = BUFSIZ - strm.avail_out;
if (out_size > 0) {
fwrite(out_buf, 1, out_size, f_out);
}
} while (strm.avail_out == 0);
}
// 结束压缩
do {
strm.next_out = out_buf;
strm.avail_out = BUFSIZ;
ret = lzma_code(&strm, LZMA_FINISH);
if (ret != LZMA_OK && ret != LZMA_STREAM_END) {
printf("Compression failed: %d\n", ret);
fclose(f_in);
fclose(f_out);
lzma_end(&strm);
return 1;
}
out_size = BUFSIZ - strm.avail_out;
if (out_size > 0) {
fwrite(out_buf, 1, out_size, f_out);
}
} while (strm.avail_out == 0);
// 关闭文件和释放资源
fclose(f_in);
fclose(f_out);
lzma_end(&strm);
return 0;
}
```
编译命令:`gcc -o compress compress.c -llzma`
使用示例:`./compress input.txt output.lzma`
阅读全文
相关推荐



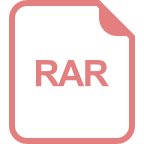





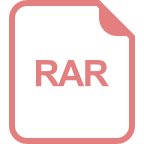

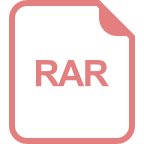
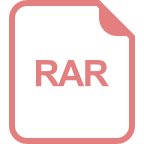



