protected override void OnPaint(PaintEventArgs e) { using (Bitmap buffer = new Bitmap(this.Width, this.Height)) { using (Graphics g = Graphics.FromImage(buffer)) { // 在缓存中绘制图像 base.OnPaint(new PaintEventArgs(g, this.ClientRectangle)); } // 将缓存中的图像渲染到控件上 e.Graphics.DrawImage(buffer, 0, 0); } }在C++/CLR 的写法
时间: 2024-02-10 12:33:49 浏览: 26
在C++/CLR中,可以使用以下代码实现同样的功能:
void MyControl::OnPaint(PaintEventArgs^ e)
{
Bitmap^ buffer = gcnew Bitmap(this->Width, this->Height);
Graphics^ g = Graphics::FromImage(buffer);
// 在缓存中绘制图像
this->OnPaint(gcnew PaintEventArgs(g, this->ClientRectangle));
// 将缓存中的图像渲染到控件上
e->Graphics->DrawImage(buffer, 0, 0);
delete g;
delete buffer;
}
相关问题
Winform中扩展Panel使之具备双缓存,防止闪屏
要使Winform中的Panel具备双缓存功能,可以通过继承Panel类并重写OnPaint方法来实现。具体步骤如下:
1. 创建一个类,继承自Panel类。
2. 重写OnPaint方法,并在方法中使用双缓存技术,将绘制的图像缓存起来,避免闪烁。
3. 在构造函数中设置ControlStyles.OptimizedDoubleBuffer样式为true,以启用双缓存。
以下是示例代码:
```
public class DoubleBufferedPanel : Panel
{
public DoubleBufferedPanel()
{
this.SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
}
protected override void OnPaint(PaintEventArgs e)
{
using (Bitmap buffer = new Bitmap(this.Width, this.Height))
{
using (Graphics g = Graphics.FromImage(buffer))
{
// 在缓存中绘制图像
base.OnPaint(new PaintEventArgs(g, this.ClientRectangle));
}
// 将缓存中的图像渲染到控件上
e.Graphics.DrawImage(buffer, 0, 0);
}
}
}
```
使用该类来创建Panel时,就可以避免闪烁问题了。
# 类似qq 快速截图 代码
以下是一个简单的 C# 快速截图的示例代码:
```
using System;
using System.Drawing;
using System.Windows.Forms;
namespace QuickScreenshot
{
public partial class MainForm : Form
{
private bool isMouseDown = false;
private Point startPoint;
public MainForm()
{
InitializeComponent();
this.DoubleBuffered = true;
this.FormBorderStyle = FormBorderStyle.None;
this.WindowState = FormWindowState.Maximized;
this.BackColor = Color.Black;
this.Opacity = 0.5;
this.TopMost = true;
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Pen pen = new Pen(Color.Red, 2);
Rectangle rect = new Rectangle(startPoint.X, startPoint.Y, Cursor.Position.X - startPoint.X, Cursor.Position.Y - startPoint.Y);
e.Graphics.DrawRectangle(pen, rect);
}
protected override void OnMouseDown(MouseEventArgs e)
{
base.OnMouseDown(e);
if (e.Button == MouseButtons.Left)
{
isMouseDown = true;
startPoint = Cursor.Position;
}
}
protected override void OnMouseMove(MouseEventArgs e)
{
base.OnMouseMove(e);
if (isMouseDown)
{
this.Invalidate();
}
}
protected override void OnMouseUp(MouseEventArgs e)
{
base.OnMouseUp(e);
if (e.Button == MouseButtons.Left)
{
isMouseDown = false;
Rectangle rect = new Rectangle(startPoint.X, startPoint.Y, Cursor.Position.X - startPoint.X, Cursor.Position.Y - startPoint.Y);
Bitmap bitmap = new Bitmap(rect.Width, rect.Height);
Graphics g = Graphics.FromImage(bitmap);
g.CopyFromScreen(rect.Left, rect.Top, 0, 0, bitmap.Size);
Clipboard.SetImage(bitmap);
this.Close();
}
}
}
}
```
这个代码实现了以下功能:
1. 点击左键开始选择截图区域,松开左键结束选择。
2. 选择区域时,会在屏幕上显示一个半透明的红色矩形框。
3. 选择完成后,将截图保存到剪贴板中,并关闭窗口。
可以根据需要对代码进行修改和优化。
相关推荐
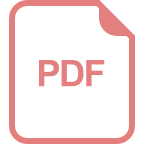
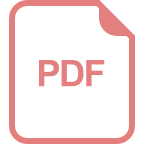











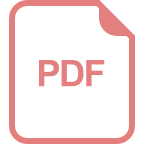
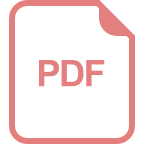