用html+JS代码写一个添加问题页面,页面分为左右两个盒子,在页面左侧盒子可以选择题型,能够实现添加单选题,多选题和问答题功能。页面右侧盒子有标题输入框、问卷说明输入框以及一个空白区域,使用左侧添加题型功
时间: 2023-08-31 20:31:27 浏览: 52
### 回答1:
能够动态向右侧空白区域添加问题。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>添加问题页面</title>
<meta charset="utf-8">
<style type="text/css">
.container {
display: flex;
flex-wrap: wrap;
width: 100%;
height: 100%;
padding: 20px;
}
.left-box {
flex: 1;
display: flex;
flex-direction: column;
padding: 20px;
background-color: #f0f0f0;
}
.right-box {
flex: 3;
display: flex;
flex-direction: column;
padding: 20px;
background-color: #fff;
}
.question-type {
margin: 10px 0;
}
.question-type label {
margin-right: 10px;
}
.question {
margin: 10px 0;
}
.question input {
margin-right: 10px;
}
</style>
</head>
<body>
<div class="container">
<div class="left-box">
<div class="question-type">
<label><input type="radio" name="question-type" value="single-choice">单选题</label>
<label><input type="radio" name="question-type" value="multi-choice">多选题</label>
<label><input type="radio" name="question-type" value="short-answer">问答题</label>
</div>
<div class="question">
<button onclick="addQuestion()">添加问题</button>
</div>
</div>
<div class="right-box">
<div class="title">
<label for="title">标题:</label>
<input type="text" id="title">
</div>
<div class="description">
<label for="description">问卷说明:</label>
<textarea id="description"></textarea>
</div>
<div class="questions">
</div>
</div>
</div>
<script type="text/javascript">
function addQuestion() {
var questionType = document.querySelector('input[name="question-type"]:checked').value;
var questionBox = document.createElement('div');
questionBox.classList.add('question-box');
var questionTitle = document.createElement('div');
questionTitle.classList.add('question-title');
if (questionType === 'single-choice') {
questionTitle.innerText = '单选题';
} else if (questionType === 'multi-choice') {
questionTitle.innerText = '多选题';
} else if (questionType === 'short-answer') {
questionTitle.innerText = '问答题';
}
questionBox.appendChild(questionTitle);
var questionContent = document.createElement('div');
questionContent.classList.add('question-content');
var questionInput = document.createElement('input');
questionInput.type = 'text';
questionInput.classList.add('question-input');
questionContent.appendChild(questionInput);
if (questionType === 'single-choice' || questionType === 'multi-choice') {
var optionBox = document.createElement('div');
optionBox.classList.add('option-box');
var optionInput = document.createElement('input');
optionInput.classList.add('option-input');
optionInput.type = 'text';
optionBox.appendChild(optionInput);
var addOptionBtn = document.createElement('button');
addOptionBtn.innerText = '+';
addOptionBtn.onclick = function() {
var optionInput = document.createElement('input');
optionInput.classList.add('option-input');
optionInput.type = 'text';
optionBox.insertBefore(optionInput, addOptionBtn);
};
optionBox.appendChild(addOptionBtn);
questionContent.appendChild(optionBox);
}
questionBox.appendChild(questionContent);
var questionsBox = document.querySelector('.questions');
questionsBox.appendChild(questionBox);
}
</script>
</body>
</html>
```
JS代码实现了添加问题和添加选项的功能,可以根据实际需求进行修改。在页面左侧选择题型后,点击“添加问题”按钮即可添加问题。问题和选项的输入框均为文本框,可以根据实际需求进行修改。
### 回答2:
可以使用HTML和JavaScript编写一个添加问题页面,页面分为左右两个盒子。在页面左侧盒子可以选择题型,并能够实现添加单选题、多选题和问答题功能。页面右侧盒子有标题输入框、问卷说明输入框以及一个空白区域,使用左侧添加题型功能。
首先,我们可以使用HTML创建页面的基本结构,包括两个盒子、标题输入框、问卷说明输入框以及空白区域。
```html
<!DOCTYPE html>
<html>
<head>
<title>添加问题页面</title>
<script src="script.js"></script>
</head>
<body>
<div class="left-box">
<h3>选择题型:</h3>
<button onclick="addSingleChoice()">添加单选题</button>
<button onclick="addMultipleChoice()">添加多选题</button>
<button onclick="addEssay()">添加问答题</button>
</div>
<div class="right-box">
<h3>标题:</h3>
<input type="text" id="title" />
<h3>问卷说明:</h3>
<textarea id="description"></textarea>
<h3>问题:</h3>
<div id="questions"></div>
</div>
</body>
</html>
```
接下来,我们使用JavaScript编写相应的函数,实现添加单选题、多选题和问答题的功能。
```javascript
function addSingleChoice() {
var questionsContainer = document.getElementById("questions");
var question = document.createElement("div");
question.innerHTML = "单选题:<input type='text' /><button>删除</button>";
questionsContainer.appendChild(question);
}
function addMultipleChoice() {
var questionsContainer = document.getElementById("questions");
var question = document.createElement("div");
question.innerHTML = "多选题:<input type='text' /><button>删除</button>";
questionsContainer.appendChild(question);
}
function addEssay() {
var questionsContainer = document.getElementById("questions");
var question = document.createElement("div");
question.innerHTML = "问答题:<input type='text' /><button>删除</button>";
questionsContainer.appendChild(question);
}
```
在这段代码中,我们分别实现了添加单选题、多选题和问答题的功能。每次调用这些函数时,都会在右侧盒子的问题区域中添加一个新的题目输入框,并且包含一个删除按钮。
这样,通过选择题型并点击对应按钮,就可以在右侧盒子中添加相应类型的题目。页面左侧的盒子提供了选择题型的功能。对于每个题目,可以输入相应的问题文本,并且可以通过删除按钮来删除题目。
这样就实现了一个添加问题的页面,用户可以方便地添加单选题、多选题和问答题,并进行相关的编辑操作。
### 回答3:
一、HTML部分:
```html
<!DOCTYPE html>
<html>
<head>
<title>添加问题页面</title>
<script src="script.js"></script> <!-- 引入JS文件 -->
<style>
/* CSS样式可以自定义,根据UI设计来设置 */
.container {
display: flex;
justify-content: space-between;
}
.leftBox, .rightBox {
width: 45%;
margin: 30px;
padding: 20px;
border: 1px solid #ccc;
box-sizing: border-box;
}
textarea {
width: 100%;
height: 100px;
margin-bottom: 10px;
}
.blankArea {
width: 100%;
height: 200px;
border: 1px solid #ccc;
box-sizing: border-box;
}
</style>
</head>
<body>
<div class="container">
<div class="leftBox">
<h2>题型选择</h2>
<input type="radio" name="questionType" value="单选题">单选题<br>
<input type="radio" name="questionType" value="多选题">多选题<br>
<input type="radio" name="questionType" value="问答题">问答题<br>
<button onclick="addQuestion()">添加题目</button>
</div>
<div class="rightBox">
<h2>添加问题</h2>
<label for="questionTitle">标题:</label>
<input type="text" id="questionTitle"><br>
<label for="questionDesc">问卷说明:</label>
<textarea id="questionDesc"></textarea>
<div id="blankArea" class="blankArea"></div>
</div>
</div>
</body>
</html>
```
二、JS部分:
```javascript
function addQuestion() {
var questionType = document.querySelector('input[name="questionType"]:checked').value;
var questionTitle = document.getElementById('questionTitle').value;
var questionDesc = document.getElementById('questionDesc').value;
var blankArea = document.getElementById('blankArea');
if (questionType === "单选题") {
var radioQuestion = document.createElement('div');
radioQuestion.innerHTML = '<h3>单选题:' + questionTitle + '</h3>' + '<p>说明:' + questionDesc + '</p>' + '<ul><li>选项1</li><li>选项2</li><li>选项3</li></ul>';
blankArea.appendChild(radioQuestion);
} else if (questionType === "多选题") {
var checkboxQuestion = document.createElement('div');
checkboxQuestion.innerHTML = '<h3>多选题:' + questionTitle + '</h3>' + '<p>说明:' + questionDesc + '</p>' + '<ul><li>选项1</li><li>选项2</li><li>选项3</li></ul>';
blankArea.appendChild(checkboxQuestion);
} else if (questionType === "问答题") {
var textareaQuestion = document.createElement('div');
textareaQuestion.innerHTML = '<h3>问答题:' + questionTitle + '</h3>' + '<p>说明:' + questionDesc + '</p>' + '<textarea></textarea>';
blankArea.appendChild(textareaQuestion);
}
}
```
以上代码实现了一个简单的添加问题页面,左侧盒子提供了题型选择的选项,右侧盒子包含了标题输入框、问卷说明输入框以及一个空白区域。通过选择左侧盒子中的题型并点击“添加题目”按钮,可以动态地在右侧的空白区域中添加相应题型的问题,并显示题目的标题、说明和选项(或空白输入框)。
相关推荐
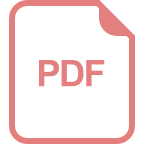
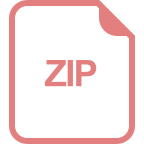
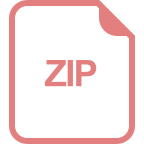














