在Linux编写C代码或者汇编代码,操作串口,网卡,音卡等设备,至少操作两种设备,给出代码
时间: 2024-01-02 12:05:12 浏览: 113
好的,下面我将为您提供两种设备的操作代码,分别是串口和网卡。
1. 操作串口
在Linux中,串口设备一般被挂载在/dev/tty*下,可以使用标准C库函数open()打开串口设备,使用read()和write()函数读写串口数据。下面是一个简单的串口读写代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main(int argc, char *argv[])
{
int fd;
char buf[256];
struct termios tio;
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
exit(EXIT_FAILURE);
}
// 配置串口参数
tcgetattr(fd, &tio);
cfmakeraw(&tio);
cfsetspeed(&tio, B9600);
tcsetattr(fd, TCSANOW, &tio);
// 读写数据
write(fd, "hello world", 11);
read(fd, buf, sizeof(buf));
printf("received: %s\n", buf);
// 关闭串口设备
close(fd);
return 0;
}
```
上面的代码中使用了标准C库函数open()打开串口设备,使用tcgetattr()和tcsetattr()函数配置串口参数,使用write()和read()函数进行读写操作。
2. 操作网卡
在Linux中,网卡设备一般被挂载在/dev/net下,可以使用标准C库函数socket()创建一个套接字,使用ioctl()函数对网卡进行配置,使用send()和recv()函数发送和接收数据。下面是一个简单的网卡发送和接收数据的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <linux/if.h>
#include <linux/if_ether.h>
int main(int argc, char *argv[])
{
int sock;
char buf[ETH_FRAME_LEN];
struct ifreq ifr;
struct sockaddr_ll sll;
// 创建套接字
sock = socket(PF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sock < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
// 配置网卡
strcpy(ifr.ifr_name, "eth0");
ioctl(sock, SIOCGIFINDEX, &ifr);
memset(&sll, 0, sizeof(sll));
sll.sll_family = AF_PACKET;
sll.sll_ifindex = ifr.ifr_ifindex;
// 发送数据
memset(buf, 0, sizeof(buf));
strcpy(buf, "hello world");
sendto(sock, buf, strlen(buf), 0, (struct sockaddr *)&sll, sizeof(sll));
// 接收数据
memset(buf, 0, sizeof(buf));
recv(sock, buf, sizeof(buf), 0);
printf("received: %s\n", buf);
// 关闭套接字
close(sock);
return 0;
}
```
上面的代码中使用了标准C库函数socket()创建一个套接字,使用ioctl()函数对网卡进行配置,使用sendto()和recv()函数发送和接收数据。
阅读全文
相关推荐
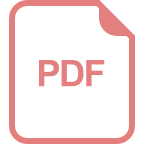
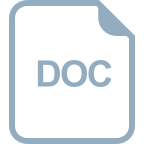
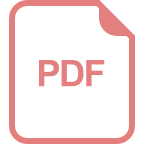
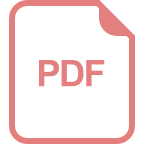
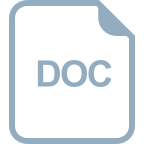
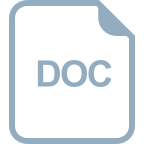
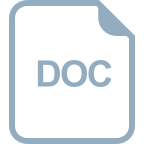
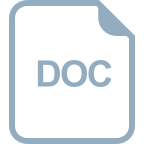
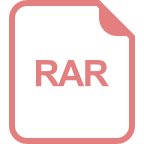