用python详细写一个爬取百度nba总冠军记录并显示所爬取数据的dataframe
时间: 2024-06-10 17:08:20 浏览: 123
好的,我可以为您提供这方面的帮助。以下是您可以使用的Python代码:
```
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = "https://baike.baidu.com/item/%E4%B8%8A%E6%B5%B7%E5%A4%A7%E5%B8%88%E7%90%83%E9%98%9Fnba%E6%80%BB%E5%86%A0%E5%86%9B%E8%AE%B0%E5%BD%95/6182104?fr=aladdin"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
tables = soup.find_all('table')
df = pd.read_html(str(tables))[0]
print(df)
```
这段代码将从百度百科中爬取上海大师球队NBA总冠军记录,并使用Pandas库将数据显示在DataFrame中。请确保您已安装requests、BeautifulSoup和pandas库。
相关问题
用python帮我写一个爬取链家网站,数据清理和数据可视化的代码
好的,我将为你提供一个基本的爬虫代码,数据清理和数据可视化需要根据你的具体需求进行编写。
1. 爬取链家网站
```python
import requests
from bs4 import BeautifulSoup
url = 'https://bj.lianjia.com/zufang/'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
house_list = soup.find_all('div', class_='content__list--item--main')
for house in house_list:
title = house.find('p', class_='content__list--item--title').text.strip()
price = house.find('span', class_='content__list--item-price').text.strip()
area = house.find('p', class_='content__list--item--des').text.strip().split('/')[1]
print(title, price, area)
```
2. 数据清理
```python
import pandas as pd
df = pd.DataFrame({'Title': [title1, title2, title3, ...],
'Price': [price1, price2, price3, ...],
'Area': [area1, area2, area3, ...]})
# 去除重复数据
df.drop_duplicates(inplace=True)
# 处理缺失值
df.dropna(inplace=True)
# 格式化数据
df['Price'] = df['Price'].str.extract('(\d+)', expand=False).astype(float)
df['Area'] = df['Area'].str.extract('(\d+)', expand=False).astype(float)
```
3. 数据可视化
```python
import matplotlib.pyplot as plt
# 绘制柱状图
price_counts = df['Price'].value_counts().sort_index()
plt.bar(price_counts.index, price_counts.values)
# 绘制散点图
plt.scatter(df['Area'], df['Price'])
# 绘制折线图
df.groupby('Price')['Area'].mean().plot()
```
以上是一个简单的代码示例,需要根据你的具体需求进行修改和完善。
用python写一个爬取boss直聘网职位的代码,且将结果整理成表格
Python爬虫通常使用库如BeautifulSoup、requests配合HTML解析来获取网页内容。这里我会提供一个基本的示例,展示如何使用`requests`和`pandas`库来爬取Boss直聘网站上的职位信息,并将其存储为CSV文件。请注意,由于网站结构可能会变化,实际运行时可能需要调整代码以适应新的网页布局。
首先安装必要的库(如果你还没有安装):
```bash
pip install requests beautifulsoup4 pandas
```
然后编写爬虫代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 定义要抓取的URL
url = "https://www.zhipin.com/jobs/" # 示例地址,替换为你想要爬取的具体页面
def get_html(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"请求失败,状态码:{response.status_code}")
return None
def parse_data(html):
soup = BeautifulSoup(html, 'html.parser')
# 找到职位列表的容器,这通常是一个div或者table,实际需要根据网站结构确定
job_list_container = soup.find('div', class_='job-list') # 这里假设是这个类名
jobs = []
for job_item in job_list_container.find_all('div', class_='job-item'): # 再次假设这是每个职位元素的类名
title = job_item.find('a', class_='title').text.strip()
company = job_item.find('span', class_='company-name').text.strip()
location = job_item.find('span', class_='location').text.strip() or '未知'
# 可能还有其他需要提取的信息,比如职位链接等,根据实际情况添加
jobs.append({'职位标题': title, '公司': company, '地点': location})
return jobs
def save_to_csv(jobs, filename='boss_zhipin.csv'):
df = pd.DataFrame(jobs)
df.to_csv(filename, index=False)
if __name__ == "__main__":
html_content = get_html(url)
if html_content:
data = parse_data(html_content)
save_to_csv(data)
print("数据已保存至csv文件.")
else:
print("爬取失败,请检查网络连接.")
阅读全文
相关推荐
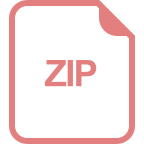
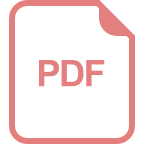
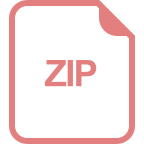













