# 读取数据集 data = pd.read_csv('./ebs/waveform-5000.csv') epsilon = 1e-10 # 去除第一行数据(属性名称) data = data.iloc[1:] # 提取属性列和类别列 X = data.iloc[:, :-1].values.astype(float) #x表示属性 y_true = data.iloc[:, -1].values #y表示类别,最后一列 # 数据标准化 scaler = MinMaxScaler(feature_range=(0, 1)) X_scaled = scaler.fit_transform(X) # 初始化NMF模型 n_components = range(2, 20) # 不同的n_components值 silhouette_scores = [] # 存储每个n_components的轮廓系数 best_silhouette_score = -1 best_n_components = -1 # 对不同的n_components进行迭代 for n in n_components: nmf = NMF(n_components=n) features = nmf.fit_transform(X_scaled) labels = nmf.transform(X_scaled).argmax(axis=1) # labels2 = nmf.components_.argmax(axis=1) # 根据聚类结果计算轮廓系数 # silhouette_avg = silhouette_score(X_scaled, labels) silhouette_avg = calinski_harabasz_score(X_scaled, labels) silhouette_scores.append(silhouette_avg) print(f"n_components={n}: Silhouette Score = {silhouette_avg}") # 选择最佳的n_components if silhouette_avg > best_silhouette_score: best_silhouette_score = silhouette_avg best_n_components = n print(f"best n_components = {best_n_components}") # 绘制得分图 plt.plot(n_components, silhouette_scores, marker='o') plt.title("NMF Clustering Performance") plt.xlabel("n_components") plt.ylabel("Silhouette Score") plt.show() print(f"best n_components = {best_n_components}") print(f"best Silhouette Score = {best_silhouette_score}") # 使用最佳的n_components进行聚类 best_nmf = NMF(n_components=best_n_components) best_features = best_nmf.fit_transform(X_scaled) # labels = best_nmf.components_.argmax(axis=1) labels = best_nmf.transform(X_scaled).argmax(axis=1) # 使用PCA进行降维和可视化 pca = PCA(n_components=2) X_pca = pca.fit_transform(X_scaled) # 可视化聚类结果 plt.scatter(X_pca[:, 0], X_pca[:, 1], c=labels) plt.title(f"NMF Clustering (n_components={best_n_components}) with PCA Visualization") plt.xlabel("Principal Component 1") plt.ylabel("Principal Component 2") plt.show()中文解析代码流程和步骤
时间: 2024-04-04 21:35:10 浏览: 93
这段代码实现了对一个数据集进行聚类分析的过程,具体流程如下:
1. 读取数据集
使用pandas库中的read_csv函数读取csv格式的数据文件,存储为data变量。
2. 数据预处理
去除第一行数据(属性名称),并将属性列和类别列分别存储为X和y_true变量。使用MinMaxScaler对属性列进行归一化处理,存储为X_scaled变量。
3. 初始化NMF模型
定义一个range(2,20)的n_components值列表,表示不同的聚类簇数。使用NMF类初始化模型,并对不同的n_components进行迭代训练。对于每个n_components,使用fit_transform函数计算特征矩阵features和标签矩阵labels。使用calinski_harabasz_score函数计算聚类结果的轮廓系数,并将结果存储在silhouette_scores列表中。
4. 选择最佳的n_components
对于每个n_components,比较其对应的轮廓系数与当前最高轮廓系数,如果更高则更新最高轮廓系数和最佳的n_components值。
5. 聚类分析
使用最佳的n_components值重新训练NMF模型,得到特征矩阵best_features和标签矩阵labels。使用PCA进行降维,将属性列降为二维,存储为X_pca变量。使用散点图可视化聚类结果,横坐标和纵坐标分别为X_pca的两个主成分,颜色表示不同的聚类簇。
总之,这段代码展示了一个完整的数据聚类分析过程,包括数据预处理、模型训练、结果评估和可视化等步骤。
相关问题
指针是如何运用的 auto ebits = ebs.begin(); while (ebits != ebs.end()) { //敌机子弹和主机碰撞模拟,HP-- if (RectDuangRect((*ebits)->GetRect(), hero.GetRect())) { delete(*ebits); ebits = ebs.erase(ebits); HP--; if (HP == 0) is_play = false; RECT a = hero.GetRect(); putimg(NULL, a.left, a.top, &sbombimg); break; }
在上述代码中,指针的运用如下:
1. 首先,使用`auto`关键字声明了一个名为`ebits`的变量,并将其初始化为`ebs`容器的起始迭代器。
2. 使用`while`循环遍历`ebs`容器中的元素,直到`ebits`迭代器等于`ebs.end()`,即到达容器的末尾。
3. 在循环中,通过解引用指针迭代器`(*ebits)`获取指向敌机子弹对象的指针,并调用其成员函数`GetRect()`获取敌机子弹的矩形区域。
4. 利用函数`RectDuangRect()`判断敌机子弹的矩形区域与主机的矩形区域是否相交,如果相交则进行碰撞模拟。
5. 如果发生碰撞,首先删除敌机子弹对象,通过`delete`关键字释放内存。
6. 调用容器的`erase()`函数删除指向该敌机子弹对象的迭代器,并返回指向下一个元素的迭代器。
7. 主机的HP减少1,如果HP减少到0,将`is_play`标志设置为`false`,表示游戏结束。
8. 获取主机的矩形区域,并使用函数`putimg()`在该区域绘制爆炸效果。
9. 使用`break`语句退出循环,只处理第一个碰撞的敌机子弹。
Apply the NMF to electrical brain signals data clustering. If possible, please give the visualization results (or any other analytical results) for clustering, where the dataset file can be downloaded from the ***\*‘./dataset/ebs/waveform-5000.csv’\****.
Sure, I can help you with that. Here's an example code to apply NMF to the electrical brain signals data clustering using the dataset file 'waveform-5000.csv':
```python
import pandas as pd
from sklearn.decomposition import NMF
import matplotlib.pyplot as plt
# Load the dataset
data = pd.read_csv('./dataset/ebs/waveform-5000.csv', header=None)
# Apply NMF with 5 components
nmf = NMF(n_components=5, init='random', random_state=0)
W = nmf.fit_transform(data)
H = nmf.components_
# Visualize the clustering results
plt.scatter(W[:, 0], W[:, 1], c=W.argmax(axis=1), cmap='viridis')
plt.xlabel('Component 1')
plt.ylabel('Component 2')
plt.show()
```
In this code, we first load the dataset using pandas and then apply NMF with 5 components. We then visualize the clustering results using a scatter plot, where the x-axis represents the first component and the y-axis represents the second component. The color of each data point represents the cluster membership, which is determined by the component with the highest weight for that data point.
Please note that the above code is just an example and you may need to tune the number of components or try different initialization methods to get better clustering results.
阅读全文
相关推荐
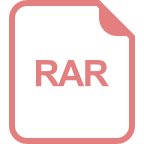
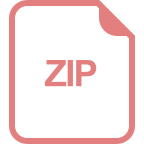
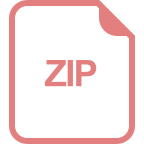
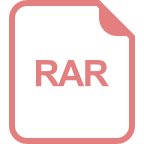






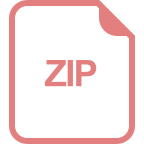
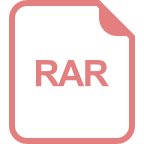