tensorboard可视化实例
时间: 2023-09-11 14:04:02 浏览: 44
TensorBoard是TensorFlow的一个可视化工具,可以帮助我们更好地理解和调试TensorFlow模型。
下面是一个简单的TensorBoard可视化实例:
1.导入必要的库和数据集
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
```
2.定义一个简单的三层神经网络模型
```python
with tf.name_scope("input"):
x = tf.placeholder(tf.float32, [None, 784], name="x-input")
y = tf.placeholder(tf.float32, [None, 10], name="y-input")
with tf.name_scope("layer"):
with tf.name_scope("weights"):
W = tf.Variable(tf.zeros([784, 10]), name="W")
with tf.name_scope("biases"):
b = tf.Variable(tf.zeros([10]), name="b")
with tf.name_scope("softmax"):
y_pred = tf.nn.softmax(tf.matmul(x, W) + b)
```
3.定义交叉熵损失函数和优化器
```python
with tf.name_scope("loss"):
cross_entropy = tf.reduce_mean(-tf.reduce_sum(y * tf.log(y_pred), reduction_indices=[1]))
tf.summary.scalar("loss", cross_entropy)
with tf.name_scope("train"):
train_step = tf.train.GradientDescentOptimizer(0.5).minimize(cross_entropy)
```
4.定义准确率计算
```python
with tf.name_scope("accuracy"):
correct_prediction = tf.equal(tf.argmax(y_pred, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
tf.summary.scalar("accuracy", accuracy)
```
5.合并所有的summary
```python
merged_summary = tf.summary.merge_all()
```
6.定义Session和FileWriter来保存summary
```python
with tf.Session() as sess:
writer = tf.summary.FileWriter("logs/", sess.graph)
sess.run(tf.global_variables_initializer())
for i in range(1000):
batch_xs, batch_ys = mnist.train.next_batch(100)
_, summary = sess.run([train_step, merged_summary], feed_dict={x: batch_xs, y: batch_ys})
writer.add_summary(summary, i)
print(sess.run(accuracy, feed_dict={x: mnist.test.images, y: mnist.test.labels}))
```
7.在终端中运行以下命令以启动TensorBoard
```bash
tensorboard --logdir=logs/
```
8.在浏览器中打开http://localhost:6006/,即可看到TensorBoard的可视化结果。
这个例子中,我们使用TensorBoard来可视化模型的损失函数和准确率的变化,以及模型结构的图形化展示。通过TensorBoard的可视化,我们可以更加直观地了解模型的训练过程和性能表现。
相关推荐
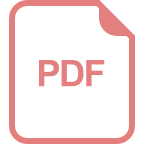
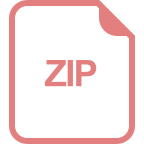














