Linux下用C语言编写一个应用程序,命名为b。这个程序不断地输出如下行:Those output come from child,[系统时间] 。
时间: 2024-02-05 14:13:33 浏览: 85
以下是一个基本的C语言程序,可以实现你所需的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
int main() {
pid_t pid;
time_t t;
while(1) {
pid = fork();
if (pid == 0) { // child process
t = time(NULL);
printf("Those output come from child, %s", ctime(&t));
exit(0);
} else if (pid > 0) { // parent process
wait(NULL);
sleep(1);
} else { // fork failed
printf("Fork failed.\n");
exit(1);
}
}
return 0;
}
```
程序中使用了fork函数来创建子进程,然后在子进程中获取系统时间并输出。父进程等待子进程结束后再休眠1秒钟,然后再创建下一个子进程。这样就可以不断地输出类似的行了。
相关问题
Linux下用C语言编写一个应用程序,命名为b。这个程序不断地输出如下行:Those output come from child,[系统时间] 。另外写一个应用程序,命名为a。这个程序创建一个子进程,执行b。
好的,我来回答你的问题。
首先,我们需要在Linux下使用C语言编写应用程序b。下面是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
int main() {
while (1) {
time_t t = time(NULL);
printf("Those output come from child,[%s]\n", ctime(&t));
sleep(1); // 每隔1秒输出一次
}
return 0;
}
```
这个程序会不断地输出类似于"Those output come from child,[系统时间]"的信息,并且每隔1秒输出一次。接下来,我们需要编写应用程序a来创建子进程并执行b。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork(); // 创建子进程
if (pid == 0) {
// 子进程执行应用程序b
execl("./b", "b", NULL);
exit(0); // 子进程执行完毕后退出
} else if (pid > 0) {
// 父进程等待子进程执行完毕
waitpid(pid, NULL, 0);
} else {
printf("Failed to create child process!\n");
exit(1);
}
return 0;
}
```
这个程序会创建一个子进程,并在子进程中执行应用程序b。父进程会等待子进程执行完毕后退出。注意,需要把应用程序b的可执行文件命名为"b"并放在同一目录下。
希望这个回答能够解决你的问题!
用C语言编写一个应用程序,命名为b。这个程序不断地输出如下行:Thoseoutputcomefromchild,[系统时间]。另外写一个应用程序,命名为a。这个程序创建一个子进程,执行b。
回答您的问题,好的,我明白了!针对您的问题,以下是您所需要的C语言程序:
程序b:
```c
#include <stdio.h>
#include <time.h>
int main() {
while(1) {
time_t now;
time(&now);
printf("Those output come from child, [%s]", ctime(&now));
sleep(1); // 暂停1秒钟
}
return 0;
}
```
程序a:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork(); // 创建子进程
if (pid < 0) {
printf("创建子进程失败\n");
exit(1);
} else if (pid == 0) {
execlp("./b", "b", NULL); // 执行程序b
} else {
wait(NULL); // 等待子进程结束
printf("子进程已结束\n");
}
return 0;
}
```
程序a通过调用fork()函数创建子进程,在子进程中使用execlp()函数执行程序b,而父进程则等待子进程结束。程序b会不断地输出一行文字,其中包含当前系统时间。
阅读全文
相关推荐
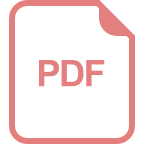
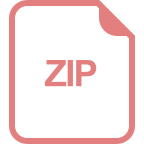
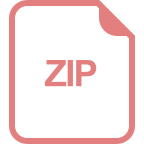
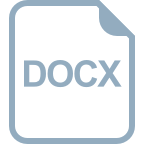
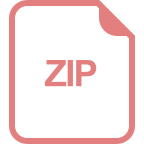
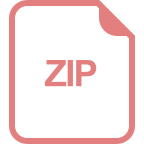
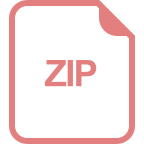
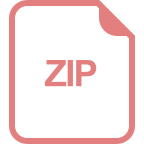