java分片读写文件
时间: 2023-07-08 18:19:33 浏览: 82
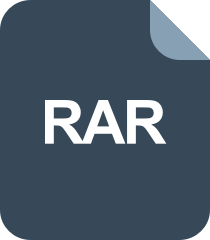
java读写文件
在Java中,对于大文件的读写,可以使用分片读写的方式来提高效率和降低内存消耗。以下是一个简单的分片读写文件的示例代码:
```java
import java.io.*;
public class FileSplitter {
private static final int BUFFER_SIZE = 1024 * 1024; // 每次读取的字节数
public static void split(String inputPath, String outputPath, int chunkSize) throws IOException {
File inputFile = new File(inputPath);
long fileSize = inputFile.length();
int numChunks = (int) Math.ceil((double) fileSize / chunkSize);
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(inputFile))) {
byte[] buffer = new byte[BUFFER_SIZE];
for (int i = 0; i < numChunks; i++) {
int bytesRead = 0;
int bytesToRead = chunkSize;
if (i == numChunks - 1) {
// 最后一片可能不足chunkSize字节
bytesToRead = (int) (fileSize - i * chunkSize);
}
try (BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(outputPath + ".part" + i))) {
while (bytesRead < bytesToRead) {
int bytes = bis.read(buffer, 0, Math.min(BUFFER_SIZE, bytesToRead - bytesRead));
if (bytes < 0) {
break;
}
bytesRead += bytes;
bos.write(buffer, 0, bytes);
}
}
}
}
}
public static void merge(String[] inputPaths, String outputPath) throws IOException {
try (BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(outputPath))) {
for (String inputPath : inputPaths) {
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(inputPath))) {
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead = 0;
while ((bytesRead = bis.read(buffer)) != -1) {
bos.write(buffer, 0, bytesRead);
}
}
}
}
}
}
```
其中,`split()`方法用于将一个大文件分成多个小文件,`merge()`方法用于将多个小文件合并成一个大文件。`chunkSize`参数指定每个小文件的大小,可以根据需要进行调整。在读取文件时,使用`BufferedInputStream`和`BufferedOutputStream`进行缓存,以提高效率。需要注意的是,在使用完输入输出流后,需要调用`close()`方法来关闭流,或使用`try-with-resources`语句来自动关闭流。
阅读全文
相关推荐
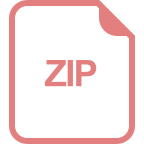
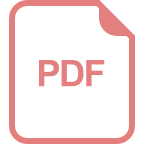
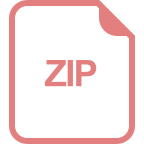
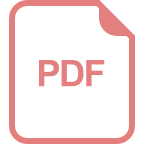
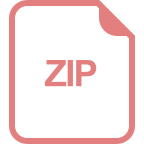
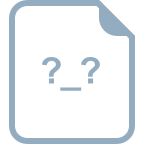
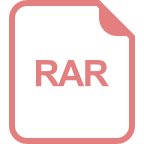
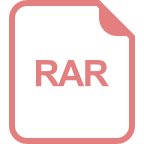
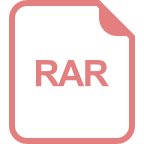
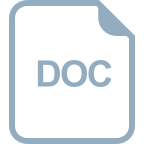
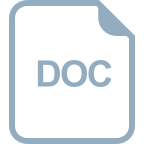
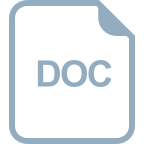
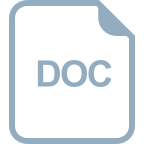
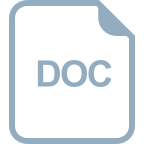
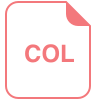

