使用c++中的继承与派生,class类来编写模拟某单位的人事管理。人事信息主要包括:员工编号、所在部门、职务、薪资等级、薪资、联系方式等内容;部门信息主要包括:部门编号、部门名称等内容。完成以下操作:实现员工信息、部门信息的添加、修改、删除和查询。
时间: 2023-07-10 22:33:37 浏览: 75
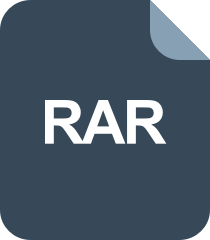
简单的员工信息管理--实现查询、添加、修改、更新等操作
好的,根据您的要求,我可以给您提供一份C++代码,实现人事管理系统的基本功能。请注意,以下代码仅供参考,您可以根据自己的需求进行修改和完善。
首先,我们需要定义两个类,一个是员工类,一个是部门类。代码如下:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Employee {
public:
Employee(int id, string dept, string position, int salary_level, double salary, string contact_info) :
m_id(id), m_dept(dept), m_position(position), m_salary_level(salary_level), m_salary(salary), m_contact_info(contact_info) {}
int get_id() const { return m_id; }
string get_dept() const { return m_dept; }
string get_position() const { return m_position; }
int get_salary_level() const { return m_salary_level; }
double get_salary() const { return m_salary; }
string get_contact_info() const { return m_contact_info; }
void set_dept(string dept) { m_dept = dept; }
void set_position(string position) { m_position = position; }
void set_salary_level(int salary_level) { m_salary_level = salary_level; }
void set_salary(double salary) { m_salary = salary; }
void set_contact_info(string contact_info) { m_contact_info = contact_info; }
private:
int m_id;
string m_dept;
string m_position;
int m_salary_level;
double m_salary;
string m_contact_info;
};
class Department {
public:
Department(int id, string name) : m_id(id), m_name(name) {}
int get_id() const { return m_id; }
string get_name() const { return m_name; }
void set_name(string name) { m_name = name; }
private:
int m_id;
string m_name;
};
```
接下来,我们需要定义一个管理类,用于管理员工和部门信息的添加、修改、删除和查询。代码如下:
```cpp
class HRSystem {
public:
void add_employee(int id, string dept, string position, int salary_level, double salary, string contact_info) {
Employee emp(id, dept, position, salary_level, salary, contact_info);
m_employees.push_back(emp);
}
void add_department(int id, string name) {
Department dept(id, name);
m_departments.push_back(dept);
}
void update_employee(int id, string dept, string position, int salary_level, double salary, string contact_info) {
for (auto& emp : m_employees) {
if (emp.get_id() == id) {
emp.set_dept(dept);
emp.set_position(position);
emp.set_salary_level(salary_level);
emp.set_salary(salary);
emp.set_contact_info(contact_info);
break;
}
}
}
void update_department(int id, string name) {
for (auto& dept : m_departments) {
if (dept.get_id() == id) {
dept.set_name(name);
break;
}
}
}
void delete_employee(int id) {
for (auto it = m_employees.begin(); it != m_employees.end(); ++it) {
if (it->get_id() == id) {
m_employees.erase(it);
break;
}
}
}
void delete_department(int id) {
for (auto it = m_departments.begin(); it != m_departments.end(); ++it) {
if (it->get_id() == id) {
m_departments.erase(it);
break;
}
}
}
void print_employee(int id) const {
for (auto& emp : m_employees) {
if (emp.get_id() == id) {
cout << "ID: " << emp.get_id() << endl;
cout << "Department: " << emp.get_dept() << endl;
cout << "Position: " << emp.get_position() << endl;
cout << "Salary Level: " << emp.get_salary_level() << endl;
cout << "Salary: " << emp.get_salary() << endl;
cout << "Contact Info: " << emp.get_contact_info() << endl;
break;
}
}
}
void print_department(int id) const {
for (auto& dept : m_departments) {
if (dept.get_id() == id) {
cout << "ID: " << dept.get_id() << endl;
cout << "Name: " << dept.get_name() << endl;
break;
}
}
}
private:
vector<Employee> m_employees;
vector<Department> m_departments;
};
```
最后,我们可以在主函数中调用这些函数,完成人事管理系统的基本功能。代码如下:
```cpp
int main() {
HRSystem hr_system;
// 添加员工和部门信息
hr_system.add_employee(1001, "Sales", "Sales Manager", 3, 5000.0, "sales@company.com");
hr_system.add_employee(1002, "Marketing", "Marketing Manager", 3, 5000.0, "marketing@company.com");
hr_system.add_employee(1003, "HR", "HR Manager", 3, 5000.0, "hr@company.com");
hr_system.add_department(1, "Sales");
hr_system.add_department(2, "Marketing");
hr_system.add_department(3, "HR");
// 修改员工和部门信息
hr_system.update_employee(1001, "Sales", "Sales Director", 4, 6000.0, "sales_director@company.com");
hr_system.update_department(1, "Sales Department");
// 删除员工和部门信息
hr_system.delete_employee(1002);
hr_system.delete_department(3);
// 查询员工和部门信息
hr_system.print_employee(1001);
hr_system.print_department(1);
return 0;
}
```
以上就是一个简单的人事管理系统的实现,您可以根据自己的需求进行修改和完善。希望能对您有所帮助!
阅读全文
相关推荐
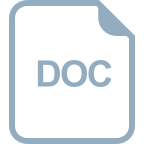
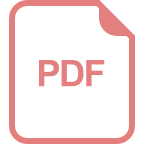
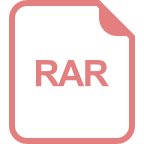
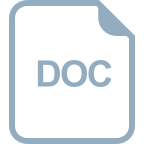
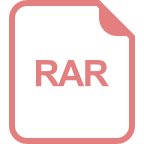
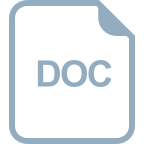
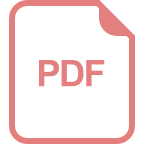
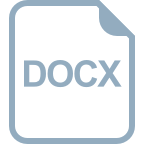