java课程设计图书管理系统代码
时间: 2024-05-28 15:06:59 浏览: 102
Java课程设计中的图书管理系统,是一个常见的小型项目,主要功能是实现对图书信息的管理。一般需要包含以下模块:图书信息管理模块、借阅管理模块、归还管理模块、用户管理模块等。
下面是一个简单的Java图书管理系统的代码示例,供参考:
```
import java.util.Scanner;
public class BookManagementSystem {
private Book[] books;
private int size;
public BookManagementSystem(int capacity) {
books = new Book[capacity];
size = 0;
}
public void addBook(Book book) {
if (size == books.length) {
System.out.println("The bookshelf is full!");
return;
}
books[size] = book;
size++;
}
public void removeBook(String bookName) {
for (int i = 0; i < size; i++) {
if (books[i].getName().equals(bookName)) {
for (int j = i; j < size - 1; j++) {
books[j] = books[j + 1];
}
books[size - 1] = null;
size--;
System.out.println("Successfully removed book: " + bookName);
return;
}
}
System.out.println("The book " + bookName + " is not found!");
}
public void borrowBook(String bookName) {
for (int i = 0; i < size; i++) {
if (books[i].getName().equals(bookName)) {
if (books[i].isBorrowed()) {
System.out.println("The book " + bookName + " is already borrowed!");
} else {
books[i].setBorrowed(true);
System.out.println("Successfully borrowed book: " + bookName);
}
return;
}
}
System.out.println("The book " + bookName + " is not found!");
}
public void returnBook(String bookName) {
for (int i = 0; i < size; i++) {
if (books[i].getName().equals(bookName)) {
if (!books[i].isBorrowed()) {
System.out.println("The book " + bookName + " is not borrowed yet!");
} else {
books[i].setBorrowed(false);
System.out.println("Successfully returned book: " + bookName);
}
return;
}
}
System.out.println("The book " + bookName + " is not found!");
}
public void listBooks() {
if (size == 0) {
System.out.println("The bookshelf is empty!");
return;
}
System.out.println("Book List:");
for (int i = 0; i < size; i++) {
System.out.println(books[i]);
}
}
public static void main(String[] args) {
BookManagementSystem bms = new BookManagementSystem(10);
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("\nMenu:");
System.out.println("1. Add a book");
System.out.println("2. Remove a book");
System.out.println("3. Borrow a book");
System.out.println("4. Return a book");
System.out.println("5. List all books");
System.out.println("6. Exit");
System.out.print("Please enter your choice: ");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("Please enter the book name: ");
String name = scanner.next();
System.out.print("Please enter the author name: ");
String author = scanner.next();
bms.addBook(new Book(name, author));
break;
case 2:
System.out.print("Please enter the book name: ");
String removeBookName = scanner.next();
bms.removeBook(removeBookName);
break;
case 3:
System.out.print("Please enter the book name: ");
String borrowBookName = scanner.next();
bms.borrowBook(borrowBookName);
break;
case 4:
System.out.print("Please enter the book name: ");
String returnBookName = scanner.next();
bms.returnBook(returnBookName);
break;
case 5:
bms.listBooks();
break;
case 6:
scanner.close();
System.exit(0);
break;
default:
System.out.println("Invalid choice!");
break;
}
}
}
}
class Book {
private String name;
private String author;
private boolean borrowed;
public Book(String name, String author) {
this.name = name;
this.author = author;
borrowed = false;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public boolean isBorrowed() {
return borrowed;
}
public void setBorrowed(boolean borrowed) {
this.borrowed = borrowed;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", borrowed=" + borrowed +
'}';
}
}
```
阅读全文
相关推荐
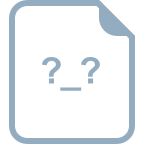
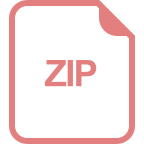
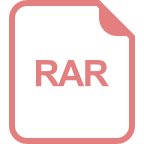
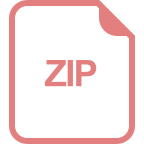
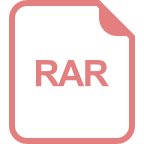
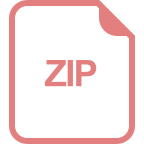
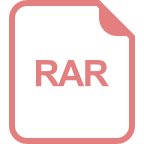
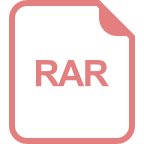
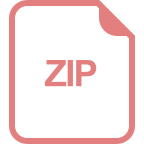
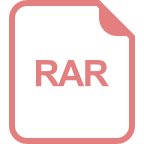
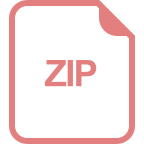
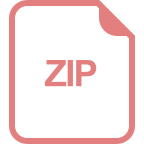
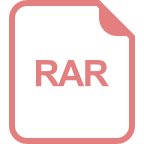
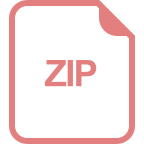
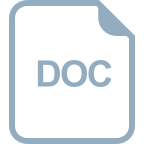
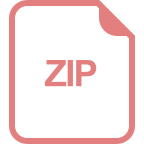