pythonTypeError: tuple object does not support item assignment
时间: 2023-07-24 11:47:22 浏览: 361
在 Python 中,元组(tuple)是不可变的对象,即一旦创建就不能修改它的值。因此,当你尝试给元组的某个元素赋值时,就会出现 “TypeError: 'tuple' object does not support item assignment” 的错误。
例如,下面的代码将尝试将元组中的第一个元素从 1 变成 2,但是会导致上述错误:
```
my_tuple = (1, 2, 3)
my_tuple[0] = 2
```
要避免这个错误,你可以考虑将元组转换为列表,修改列表中的元素,然后将其转换回元组。例如:
```
my_tuple = (1, 2, 3)
my_list = list(my_tuple)
my_list[0] = 2
my_tuple = tuple(my_list)
```
这样就可以修改元组中的元素了。但需要注意的是,这种方法会创建一个新的元组对象,而不是在原来的元组上进行修改。
相关问题
pythonTypeError: 'tuple' object does not support item assignment
这个错误通常是因为试图改变元组中的某个元素,而元组是不可变类型,无法进行修改操作。例如:
```
my_tuple = (1, 2, 3)
my_tuple[1] = 4 # 这里会抛出 'tuple' object does not support item assignment 异常
```
为了避免这个错误,您可以将元组转换成列表进行修改,然后再将列表转换回元组,或者使用新的元组替换旧的元组。例如:
```
my_tuple = (1, 2, 3)
my_list = list(my_tuple) # 将元组转换成列表
my_list[1] = 4 # 修改列表中的元素
my_tuple = tuple(my_list) # 将列表转换回元组
```
或者:
```
my_tuple = (1, 2, 3)
my_tuple = my_tuple[:1] + (4,) + my_tuple[2:] # 使用新的元组替换旧的元组
```
这样就能够避免修改元组中的元素而导致的异常。
ypeError: tuple object does not support item assignment
This error occurs when you try to assign a value to a specific index of a tuple, which is not allowed because tuples are immutable in Python. Once a tuple is created, you cannot modify its values.
For example:
```
my_tuple = (1, 2, 3)
my_tuple[0] = 4
```
This code will raise a TypeError because you are trying to assign a new value to the first index of the tuple `my_tuple`. To fix this error, you can use a list instead of a tuple, which is mutable and allows you to modify its values.
阅读全文
相关推荐
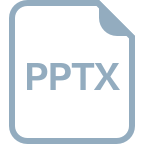
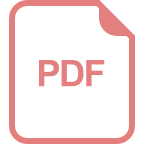
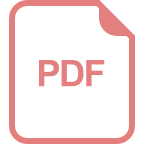










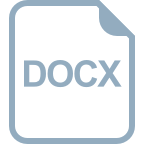