C语言,一个数组,包含eth0,eth1,eth2...ethx,bond 0,usb0,排序,eth开头的排最前面,按照eth后的数字从小到大排列,然后是bond0,最后是usb0.例如数组为{eth2,eth4,eth3,eth0,bond0,usb0,eth1}
时间: 2024-10-03 22:03:10 浏览: 14
C语言中对这种数组进行排序可以采用自定义比较函数,结合`qsort`函数。首先,你需要创建一个结构体来封装你的元素,包括网络设备名称及其分类:
```c
typedef struct {
char device[8]; // 假设设备名最多8个字符
int type; // 类型标识,如1表示eth, 2表示bond, 3表示usb
} Device;
```
然后定义一个比较函数,用于根据规则进行排序:
```c
int compareDevices(const void* a, const void* b) {
Device devA = (*(Device*)a);
Device devB = (*(Device*)b);
if (strncmp(devA.device, "eth", 3) == 0 && strncmp(devB.device, "eth", 3) == 0) {
return strcmp(devA.device + 3, devB.device + 3); // 比较eth后的数字
} else if (strcmp(devA.device, "bond0") < 0) {
return -1;
} else if (strcmp(devB.device, "bond0") > 0) {
return 1;
} else if (strcmp(devA.device, "usb0") < 0) {
return -1;
} else if (strcmp(devB.device, "usb0") > 0) {
return 1;
}
return 0;
}
```
接下来,在主程序中初始化数组并调用`qsort`进行排序:
```c
Device devices[] = {{"eth2", 1}, {"eth4", 1}, {"eth3", 1}, {"eth0", 1},
{"bond0", 2}, {"usb0", 3}, {"eth1", 1}};
int count = sizeof(devices) / sizeof(devices[0]);
// 使用自定义比较函数对数组进行排序
qsort(devices, count, sizeof(Device), compareDevices);
// 打印排序后的结果
for (int i = 0; i < count; i++) {
printf("%s, %d\n", devices[i].device, devices[i].type);
}
```
阅读全文
相关推荐
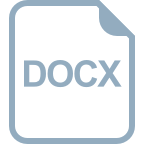
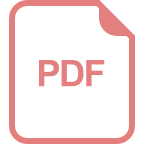
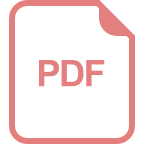
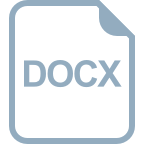
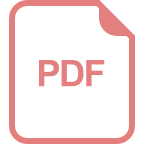
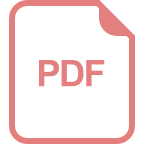
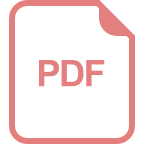
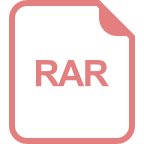
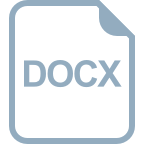
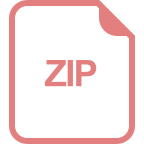
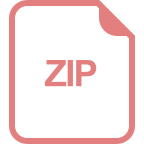
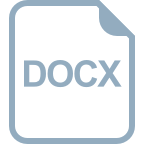
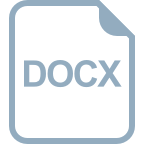