写一个贪吃蛇 python 代码
时间: 2023-08-28 22:13:38 浏览: 62
### 回答1:
好的,下面是一个简单的贪吃蛇代码:
```python
import turtle
import time
# 设置画布大小
turtle.setup(width=600, height=600)
# 设置画笔速度
turtle.speed(8)
# 画蛇的身体
def draw_snake(snake_list):
for x, y in snake_list:
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.circle(20)
# 设置蛇头初始位置
head_x = 0
head_y = 0
# 初始化蛇的身体
snake_list = [(head_x, head_y)]
draw_snake(snake_list)
# 设置食物的位置
food_x = 100
food_y = 100
# 画食物
turtle.penup()
turtle.goto(food_x, food_y)
turtle.pendown()
turtle.circle(20)
# 设置移动方向
direction = "right"
# 游戏循环
while True:
# 移动蛇头
if direction == "right":
head_x += 20
elif direction == "left":
head_x -= 20
elif direction == "up":
head_y += 20
elif direction == "down":
head_y -= 20
# 检查是否吃到食物
if head_x == food_x and head_y == food_y:
food_x = turtle.xcor()
food_y = turtle.ycor()
turtle.penup()
turtle.goto(food_x, food_y)
turtle.pendown()
turtle.circle(20)
else:
snake_list.pop(0)
# 在蛇头位置画一个新的圆形
snake_list.append((head_x, head_y))
draw_snake(snake_list)
# 设置方向
turtle.onkeypress(lambda: turtle.setheading(0), "Right")
turtle.onkeypress(lambda: turtle.setheading(180), "Left")
turtle.onkeypress(lambda: turtle.setheading(90), "Up")
turtle.onkeypress(lambda: turtle.setheading(270), "Down")
turtle.listen()
time.sleep(0.1)
```
希望这段代码对您有所帮助!
### 回答2:
贪吃蛇游戏是经典的小游戏之一,通过控制蛇的移动,吃掉食物来不断增长身体长度。下面是一个简单的贪吃蛇的Python代码示例。
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏界面的宽度和高度
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
# 定义蛇的初始化位置和身体长度
snake_pos = [[100, 50], [90, 50], [80, 50]]
snake_body = pygame.Surface((10, 10))
snake_body.fill((255, 255, 255))
# 定义食物的位置
food_pos = [random.randrange(1, 64) * 10, random.randrange(1, 48) * 10]
food_body = pygame.Surface((10, 10))
food_body.fill((255, 0, 0))
# 初始化方向
direction = 'RIGHT'
change_to = direction
# 定义游戏时钟
clock = pygame.time.Clock()
# 游戏结束标志
game_over = False
# 定义函数,控制蛇的移动
def control_snake():
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
return change_to
# 游戏主循环
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 控制蛇的移动
direction = control_snake()
# 蛇头的移动
if direction == 'UP':
snake_pos[0][1] -= 10
if direction == 'DOWN':
snake_pos[0][1] += 10
if direction == 'LEFT':
snake_pos[0][0] -= 10
if direction == 'RIGHT':
snake_pos[0][0] += 10
# 游戏结束条件判断
if snake_pos[0][0] < 0 or snake_pos[0][0] >= width or snake_pos[0][1] < 0 or snake_pos[0][1] >= height:
game_over = True
break
# 判断蛇头是否和身体碰撞
for block in snake_pos[1:]:
if snake_pos[0][0] == block[0] and snake_pos[0][1] == block[1]:
game_over = True
break
# 控制蛇身的移动
if len(snake_pos) > 1:
snake_pos[1:] = snake_pos[:-1]
# 绘制蛇和食物
screen.fill((0, 0, 0))
for pos in snake_pos:
screen.blit(snake_body, pos)
screen.blit(food_body, food_pos)
# 判断蛇是否吃到食物
if snake_pos[0][0] == food_pos[0] and snake_pos[0][1] == food_pos[1]:
food_pos = [random.randrange(1, 64) * 10, random.randrange(1, 48) * 10]
snake_pos.append([0, 0])
pygame.display.update()
clock.tick(10)
# 退出pygame
pygame.quit()
```
这段代码使用Pygame库实现了一个简单的贪吃蛇游戏。游戏界面的大小为640x480,蛇的身体长度逐渐增长。通过键盘控制方向移动蛇头,如果蛇头碰到边界或者自己的身体,游戏结束。食物会随机出现,当蛇吃到食物时,蛇的身体长度会增加,同时食物会随机重新出现在界面上。游戏的速度是通过`clock.tick(10)`来控制的,每秒钟更新10次。
### 回答3:
下面是一个简单的 Python 代码,用于实现经典的贪吃蛇游戏:
```python
import pygame
import random
# 初始化 pygame
pygame.init()
# 定义窗口的宽度和高度
width, height = 640, 480
# 创建窗口
screen = pygame.display.set_mode((width, height))
# 设置窗口标题
pygame.display.set_caption("贪吃蛇游戏")
# 定义贪吃蛇的颜色和初始位置
snake_color = (0, 255, 0)
snake_pos = [[100, 50], [90, 50], [80, 50]]
# 定义食物的颜色和初始位置
food_color = (255, 0, 0)
food_pos = [random.randint(1, (width // 10) - 1) * 10, random.randint(1, (height // 10) - 1) * 10]
# 初始化游戏分数
score = 0
# 设置游戏时钟
clock = pygame.time.Clock()
# 定义贪吃蛇的方向
direction = 'RIGHT'
# 定义移动函数
def move_snake(direction):
if direction == 'UP':
snake_head = [snake_pos[0][0], snake_pos[0][1] - 10]
elif direction == 'DOWN':
snake_head = [snake_pos[0][0], snake_pos[0][1] + 10]
elif direction == 'LEFT':
snake_head = [snake_pos[0][0] - 10, snake_pos[0][1]]
elif direction == 'RIGHT':
snake_head = [snake_pos[0][0] + 10, snake_pos[0][1]]
# 插入新的蛇头
snake_pos.insert(0, snake_head)
# 如果碰到食物,则分数加1,并重新生成食物
if snake_pos[0] == food_pos:
global score
score += 1
food_pos = [random.randint(1, (width // 10) - 1) * 10, random.randint(1, (height // 10) - 1) * 10]
else:
# 删除蛇尾
snake_pos.pop()
# 游戏循环
running = True
while running:
# 绘制背景色为黑色
screen.fill((0, 0, 0))
# 处理键盘事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and direction != 'DOWN':
direction = 'UP'
elif event.key == pygame.K_DOWN and direction != 'UP':
direction = 'DOWN'
elif event.key == pygame.K_LEFT and direction != 'RIGHT':
direction = 'LEFT'
elif event.key == pygame.K_RIGHT and direction != 'LEFT':
direction = 'RIGHT'
# 移动贪吃蛇
move_snake(direction)
# 绘制贪吃蛇
for pos in snake_pos:
pygame.draw.rect(screen, snake_color, pygame.Rect(pos[0], pos[1], 10, 10))
# 绘制食物
pygame.draw.rect(screen, food_color, pygame.Rect(food_pos[0], food_pos[1], 10, 10))
# 更新分数
font = pygame.font.Font(None, 36)
text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(text, (10, 10))
# 刷新屏幕
pygame.display.flip()
# 控制游戏帧数
clock.tick(15)
# 退出游戏
pygame.quit()
```
这个代码实现了一个简单的贪吃蛇游戏,通过方向键控制蛇的移动方向,吃到食物后分数会增加。游戏界面使用了 Pygame 库来绘制窗口、贪吃蛇和食物,并使用游戏循环来实现游戏的持续运行。
相关推荐
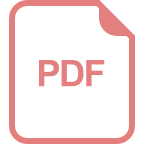
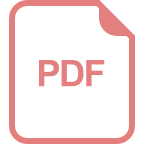
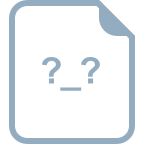
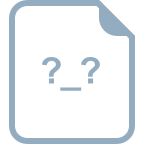
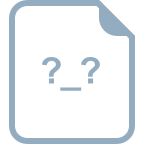
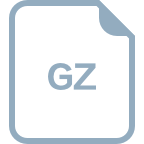
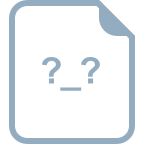
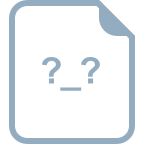